Running Terraform Locally
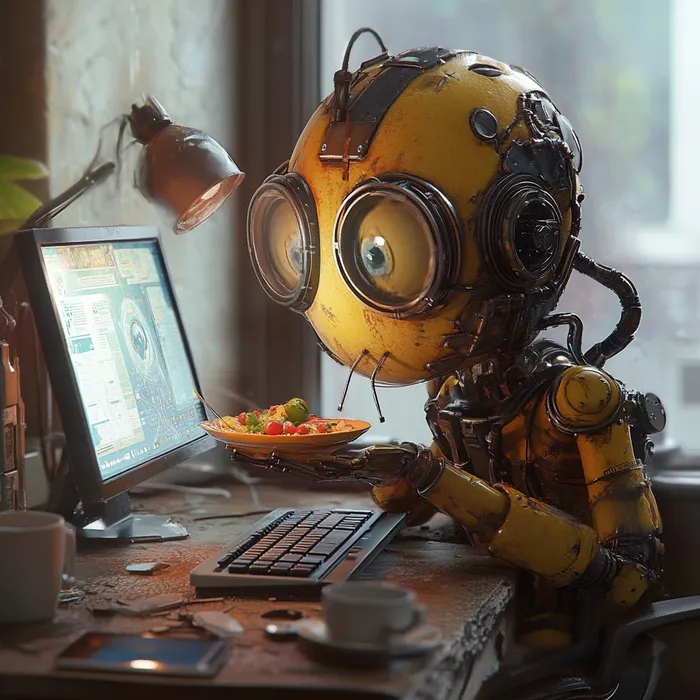
On this page
- Introduction
- What does it mean to run Terraform locally?
- When should you be running Terraform locally?
- Setting up the environment to run Terraform locally
- Running Terraform commands locally
- Commonly used commands to run Terraform locally
- Other Terraform commands
- Running Terraform locally using a shell script
- Why should you automate running Terraform commands locally using shell script?
- Difference between Terraform locals and running the Terraform CLI locally
- Conclusion
- Frequently Asked Questions
- 1. How do I install Terraform on my local machine?
- 2. How do I configure Terraform to connect to my cloud provider?
- 3. What is OpenTofu?
- 4. How do I prevent conflicts when multiple people are running Terraform locally?
Introduction
When you use Terraform on your local machine (not through a CI/CD pipeline) to set up infrastructure on a cloud provider like Azure, GCP, or AWS, this process is referred to as running Terraform locally.
In this blog, we’ll explore running various Terraform commands (like terraform fmt
, validate
, plan
, apply
, and destroy
) locally and discuss their benefits. We’ll also show how to automate these commands with a Terraform shell script. Additionally, we’ll clarify how running Terraform locally differs from using it in other environments.
What does it mean to run Terraform locally?
Running Terraform locally executes Terraform commands on your local systems and manages the infrastructure directly from your system.
To create, maintain, configure, and destroy resources using infrastructure as code, an API call is made to the cloud providers to access the infrastructure locally via Terraform.
When should you be running Terraform locally?
- Easy Setup
You only need a minimal setup while executing Terraform locally. You need to install Terraform on your local machine. Then, you must configure your Terraform code to connect to the cloud providers (AWS, GCP, Azure, etc). Now, you can create, update, and destroy resources by running Terraform locally.
- Execution Control
You do not need to commit, push, and run your CI/CD workflows when running Terraform locally. You just need to run the terraform apply
command to create the infrastructure, roll back changes, or make changes to the configuration. You will receive immediate feedback on the changes implemented, giving you complete control of how the Terraform commands are executed.
- Visibility and Debugging
You can access the logs, outputs, and state files directly with local execution. This provides complete visibility and makes the debugging process easier by helping you better understand the infrastructure code workflow.
- Simple Development and Testing Environments
If you deploy the infrastructure using Terraform locally, it does not require additional deployments or infrastructure since everything will be executed locally.
Setting up the environment to run Terraform locally
Pre-requisites
- Install Terraform
Download the Terraform binary per your operating system using the documentation for the latest version of Terraform
- Code Editor
Choose a code editor that you prefer and install it. We will be using IntelliJ in this blog
- Access to Cloud Provider
You will need an account on the cloud of your choosing to create and manage your resources. This tutorial will use the AWS cloud provider and access keys for the access.
Running Terraform commands locally
For a simple usage example, in this Terraform tutorial, we will create an aws_instance resource and experiment with Terraform commands locally.
First, we will create the input variable for instance_type in var.tf file with a default value.
variable “instance_type” { type = string // string type value default = “t2.micro”}
We will create the aws_instance resource in main.tf and use the AWS provider to build the infrastructure.
provider “aws” { region = “us-east-1” access_key = “A****************” secret_key = “U**************”}resource “aws_instance" "ec2_instance” { ami = “ami-051f8a213df8bc089” instance_type = var.instance_type}
Commonly used commands to run Terraform locally
- terraform fmt
This command is used to apply certain HCL (HashiCorp Configuration Language) language style conventions, which are canonical formats and styles with some other readability adjustments.
- terraform validate
This command checks all your configuration files to see if they are valid and consistent with the syntax. It does not use remote state or cloud providers, making it a quick and lightweight operation that can be used to maintain correct configuration files.
When you run the terraform fmt
command, you will see the file name where the formatting has been done.
The terraform validate
command displays that the configuration is valid, which means the infrastructure is configured correctly.
- terraform init
This command takes your configuration file, and installs and configures the providers and backend. It initializes the current working directory, where the command is run by preparing the necessary configuration files.
- terraform plan
This command creates the Terraform execution plan. After you run the terraform plan
command in your current working directory, it shows you the changes that will be made to the infrastructure to match the configuration state you provided. Remember, it does not make any changes to the infrastructure and only displays the changes that would take place.
When you run the terraform init
command, you can see an initialization step that downloads the plugins, configures the backend in your current working directory, and creates a lock file to record the provider details of the infrastructure code. You can see the generated Terraform configuration plan when you run the terraform plan
command. Here, the resource action indicates that it will create the aws_instance as one to add with a plus(+) sign.
- terraform apply
This command applies the changes to the infrastructure required to reach the desired configuration state. You can see the actual infrastructure changes after you run the terraform apply
command in your current working directory. It creates, updates, or destroys the infrastructure resources on the cloud.
When you run the terraform apply
command on the command line, you first see the generated plan for the infrastructure code. This displays the resource action indicating the creation of the aws_instance as one to add with a plus(+) sign using input variables. After generating the plan, it asks for your approval to apply the changes. Type yes to create the resource. You can also check it using the AWS console.
- terraform destroy
This command destroys all the Terraform-managed infrastructure resources specified in the configuration and state file. After you run the terraform destroy
command, you can see all the Terraform-managed resources that will be removed.
When you run the terraform destroy
command, you first see the generated destroy plan, which displays the resource action indicated to destroy for the aws_instance as one to destroy with a negative(-) sign. After generating the destroy plan, it asks for your approval to apply the change type yes to destroy the resource. You can see the resource deletion after giving yes as input. You can verify via the AWS console.
Other Terraform commands
- terraform import
This command imports existing infrastructure into your Terraform state. Integrating unmanaged resources into Terraform’s state without disruption is essential.
For example, we will create a dev-role as aws_iam_role in main.tf whose resources we want to import from AWS.
resource “aws_iam_role” “dev-role” {}
We will run the terraform import
command in our current working directory with the resource name and id. We will see that the state file has been updated with the resource configuration. You can verify this by running the terraform show
command.
- terraform output
This command reads and displays the output variables from your Terraform state file. These output values contain information about the resources useful for scripting or automation.
Let’s add the field that we want to get in the output using output block.
output ”aws_instance_ami_id” { value = aws_instance.ec2_instance.ami}
When we run the terraform output
command, it returns the aws_instance_ami_id we created in the example above.
Running Terraform locally using a shell script
We will run Terraform using a script that executes: terraform init
, terraform plan
, and terraform apply
. We will store the value of the Terraform plan in output and then use this output to create the aws_instance resource on the AWS public cloud.
!/bin/bash
Initialize Terraformecho "Initializing Terraform…"terraform init
Check if terraform init was successfulif [ $? -eq 0 ]; then echo "Terraform initialization successful."else echo "Terraform initialization failed." exit 1fi
Create a Terraform planecho "Creating Terraform plan…"terraform plan -out=tfplan
Check if terraform plan was successfulif [ $? -eq 0 ]; then echo "Terraform plan created successfully."else echo "Failed to create Terraform plan." exit 1fi
Ask user if they want to apply the planecho "Do you want to apply this plan? (yes/no)"read answerif [ "$answer" == "yes" ]; then # Apply the Terraform plan echo "Applying Terraform plan…" terraform apply "tfplan"
# Check if terraform apply was successful if [ $? -eq 0 ]; then echo "Terraform apply successful." else echo "Terraform apply failed." exit 1 fielse echo "Apply cancelled."fi
Now, you can run the Terraform run shell script file in its current working directory with:
$ bash ./run.sh
When the Terraform shell script is executed, it will ask for your input before proceeding with the terraform apply
command. Answer yes
to proceed.
Why should you automate running Terraform commands locally using shell script?
- Consistency
A script ensures you follow a specific order when implementing the Terraform commands, eliminating human error.
- Efficiency
When you use a script, you do not need to write the commands repeatedly, making it easier to implement frequent changes.
- Repeatability and Scalability
A script can be used repeatedly across multiple environments and is easy to modify as needed.
- Error Handling
This script includes error checks that can be included after each Terraform command, which gives immediate feedback and can be used to quickly troubleshoot, preventing any potential misconfigurations.
Difference between Terraform locals and running the Terraform CLI locally
There can be confusion when you hear the terms Terraform locals and running the Terraform CLI locally. Let’s clarify things by looking at the differences.
Parameter | Terraform Locals | Running the Terraform CLI Locally |
---|---|---|
Definition | Used to define local values in Terraform | Running a version of the Terraform CLI on your machine |
Where to define | Define local values in files like main.tf or vars.tf | Run commands in a terminal or create a script to run them |
Use case | Used to store expressions or repeated values and maintain readability | Used to deploy Terraform changes locally |
Example | locals { name = “ec2” } | terraform init , terraform plan , terraform apply |
Conclusion
Running Terraform locally is a practical and efficient approach to managing infrastructure in non-production environments. This is a greaty way to learn and test with a fast feedback loop.
While we have learned about running Terraform commands locally, if you need to integrate Terraform for a production environment using a CI/CD pipeline, consider using Terrateam.
Terrateam is a powerful Terraform and OpenTofu GitOps CI/CD solution that seamlessly integrates with GitHub, enabling teams to deliver infrastructure faster and more efficiently. With Terrateam, you can easily manage your infrastructure as code, collaborate with your team, and automate your deployment processes.
For more information, check out our docs.
Frequently Asked Questions
1. How do I install Terraform on my local machine?
You can download the binary versions of the Terraform from the official Terraform documentation
2. How do I configure Terraform to connect to my cloud provider?
You can use the provider block to support your cloud configuration with the latest version
3. What is OpenTofu?
Early on, Terraform was an open-source project, but that changed in August 2023, when HashiCorp, the company behind Terraform, made the decision to shift away from open-source licensing. OpenTofu is the world’s response to that decision. Ultimately, OpenTofu is a direct replacement for legacy Terraform. Terrateam supports both legacy Terraform and OpenTofu.
4. How do I prevent conflicts when multiple people are running Terraform locally?
Consider using a Terraform Automation and Collaboration tool (TACOS) like Terrateam.