How to apply GitFlow Workflow with Terraform
Let’s say you are working as an infrastructure engineer at an organization where multiple teams manage their cloud resources using Terraform. One team is creating a new VPC, another is changing IAM policies, and another is updating database configurations. All of these changes are happening at the same time, but without a clear process, they end up on the same branch.
When the terraform plan runs, it fails. The IAM changes depend on a VPC that hasn’t been created yet. The team adjusts the order of deployments to fix the issue. Meanwhile, a database misconfiguration goes unnoticed, leading to downtime in production. Debugging takes hours because there is no clear record of what changed and when.
This happens when teams do not follow a structured workflow. Unlike application code, infrastructure changes often rely on other resources. If they are not applied in the right order, dependencies fail, configurations are incomplete, and services may become unavailable. GitFlow helps prevent these problems by keeping changes separate, tested, and deployed in a structured way.
In this blog, we will explore how GitFlow helps manage Terraform changes by keeping updates structured, tested, and deployed in a controlled way. We’ll go through setting up GitFlow, applying it in a Terraform project, and managing feature branches, releases, and hotfixes.
What is GitFlow?
GitFlow is a branching model that helps teams manage changes in a more structured way. It defines different types of branches, making it easier to track updates, test changes, and deploy without breaking things.
In GitFlow, each branch has a specific purpose:
main
branch – Holds stable, production-ready code. Only tested changes go here.develop
branch – Used for ongoing work. All new changes are merged here before release.feature/*
branches – Created for individual changes, like adding a new VPC or updating IAM policies. These branches start from develop and are merged back when the change is ready.release/*
branches – Used to prepare for production. This is where final testing happens before merging into main.hotfix/*
branches – Used for urgent fixes in production. These branches start from main, get the fix applied, and are merged back into both main and develop.
By using GitFlow workflow, teams can work on multiple updates at the same time without interfering with each other. It keeps changes organized, reduces conflicts, and makes it easier to track what was done and when.
Use Cases for GitFlow in Infrastructure
Now, gitFlow is not just about organizing branches; it solves some real problems that DevOps teams face when they are managing their infrastructure with Terraform. It provides a structured way to track changes, isolate updates, handle production fixes, and test configurations before deployment.
Traceability and Version Control
Infrastructure changes must be tracked carefully, especially in environments where multiple teams are working on different components. With GitFlow, every change happens in a dedicated branch, making sure that the updates are properly documented. Feature branches provide a clear history of what was modified, release branches allow teams to tag versions before deployment, and hotfix branches keep urgent fixes separate from ongoing work. This structure helps teams review past changes, roll back if needed, and maintain compliance as well.
Isolating Infrastructure Updates
Making multiple changes in a single branch increases the risk of conflicts. For example, a new VPC deployment should not interfere with IAM policy updates or database changes. GitFlow enforces separation by using feature branches, making sure that each infrastructure update is developed and tested independently before merging. This prevents unexpected dependencies from breaking deployments and allows teams to work on different changes at the same time without affecting each other.
Managing Hotfixes in Production
When a misconfiguration or failure happens in production, it needs to be fixed immediately. Without a clear process, resolving a misconfigured security group or a database connection failure can be difficult and unorganized. GitFlow’s hotfix branches allow teams to create a separate branch from main
, apply the fix, and merge it back into both main
and develop
. This makes sure that the fix is deployed quickly while keeping all branches up to date, preventing future conflicts.
Testing Infrastructure Changes
Terraform changes should never go directly into production without validation. GitFlow provides a controlled process where updates are tested in isolated branches before merging. Feature branches allow teams to run terraform plan
and terraform apply
in test environments, release
branches provide a final checkpoint for verification, and hotfix
branches make sure that urgent fixes are tested before being deployed. This structured testing approach reduces deployment failures and makes sure that only stable changes reach production.
By following GitFlow, teams can track changes, avoid conflicts between different updates, apply fixes without delays, and test updates before deployment. This keeps infrastructure changes organized and reduces the chances of deployment issues.
Preparing to Use GitFlow with Terraform
Before making any changes to infrastructure, GitFlow needs to be set up properly. This makes sure that updates are tracked, tested, and merged in a structured way. The first step is to initialize a Git repository, install the git flow extension, and configure it, followed by breaking down infrastructure tasks into separate feature branches.
Setting Up the Git Repository
GitFlow is a branching model that helps teams manage changes in a more structured way. To simplify working with GitFlow, we are using the git flow extension, which you can install by following the instructions here.
Once installed, initialize a new Git repository for the Terraform project:
git flow init
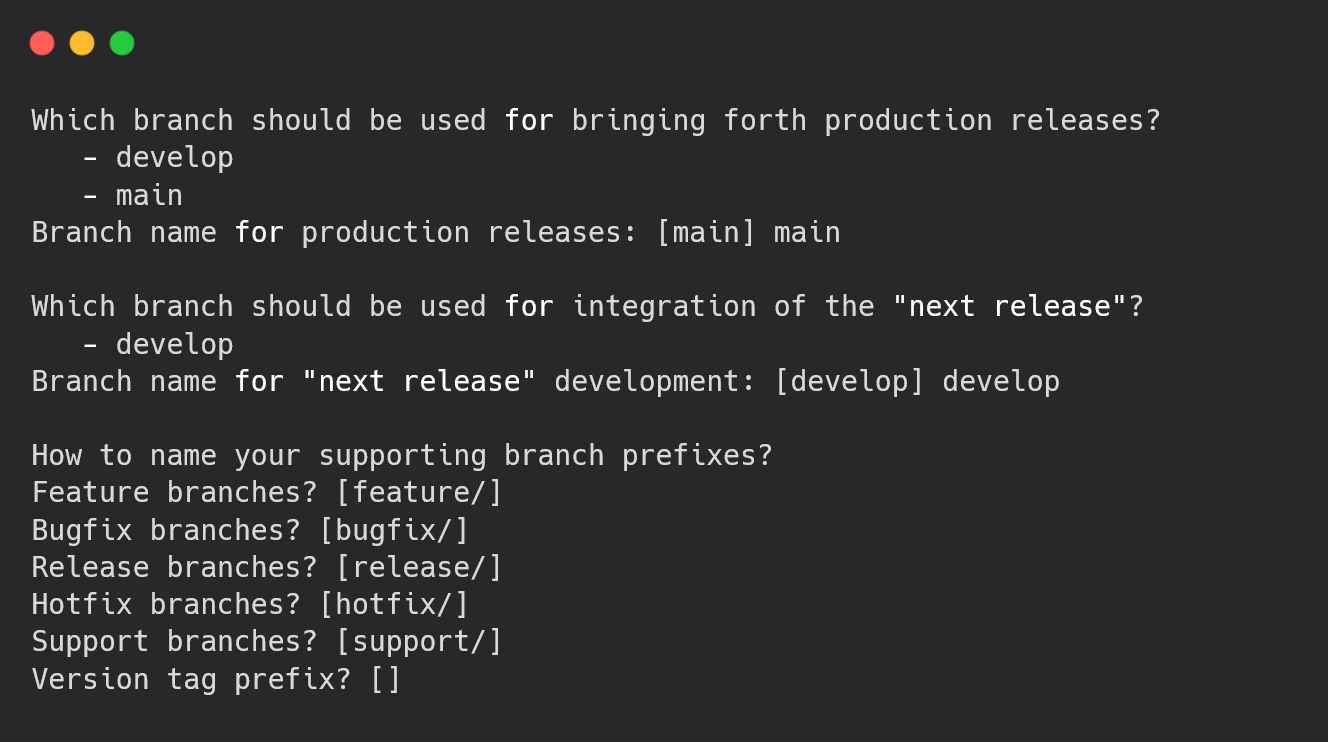
This command sets up the required branches, making sure that changes follow a structured workflow. With GitFlow in place, teams can manage infrastructure changes through dedicated branches instead of working directly in main
or develop
.
Planning Infrastructure Changes
Each update should be handled in a separate feature branch to keep changes isolated. For example:
feature/add-vpc
for creating a new VPCfeature/update-iam
for modifying IAM policiesfeature/configure-s3
for setting up S3 bucket policies
Each feature branch starts from develop
and is merged back after testing. This avoids conflicts and makes it easier to track changes.
By setting up the repository correctly and organizing updates into dedicated branches, teams can manage Terraform changes in a structured and predictable way.
Hands-On: Applying GitFlow with Terraform
Now that GitFlow is set up, we can apply it to a Terraform project. This hands-on section will go through creating a feature branch, making infrastructure changes, validating configurations, and merging updates.
Each change in Terraform should be developed in a dedicated feature branch to keep updates isolated and easy to track. In this example, we will create a feature branch to add an AWS VPC, validate it using Terraform commands, and then merge the changes back into develop
.
Starting a Feature Branch for VPC Configuration
Before making changes, we need to create a feature branch. This branch will be used to add a new AWS VPC using Terraform.
Step 1: Create a Feature Branch
Run the following command to create and switch to a new feature branch:
git flow feature start add-vpc
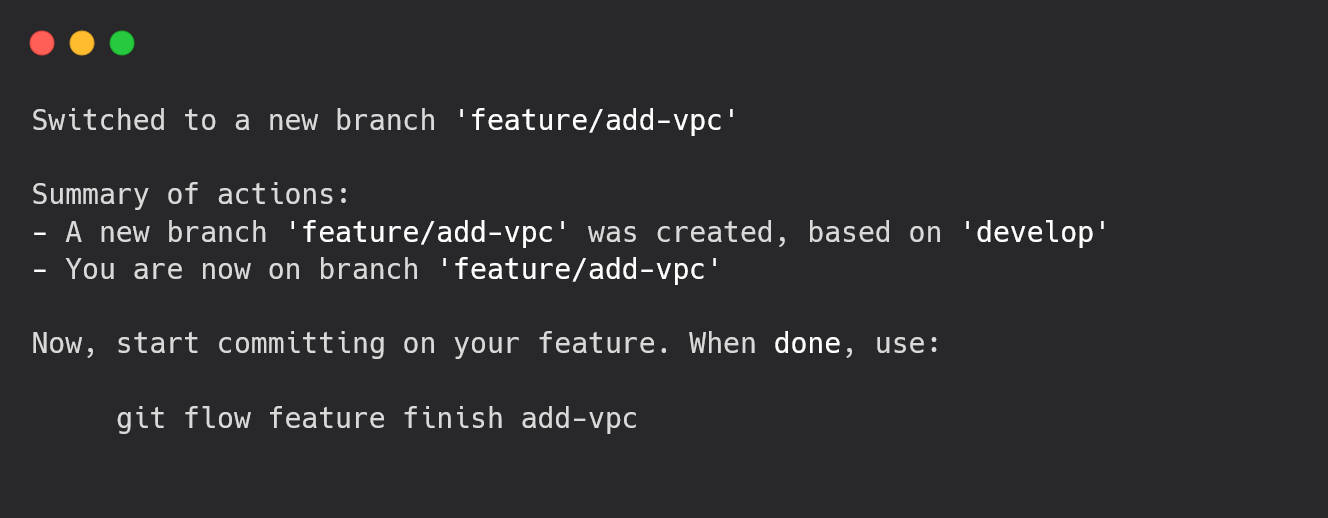
This command creates a feature/add-vpc
branch from develop
, allowing us to make changes without affecting the main codebase.
Step 2: Create Terraform Files for VPC
In the new feature branch, create a Terraform configuration file (vpc.tf) to define the AWS VPC.
provider "aws" {
region = "us-east-1"
}
resource "aws_vpc" "main_vpc" {
cidr_block = "10.0.0.0/16"
enable_dns_support = true
enable_dns_hostnames = true
tags = {
Name = "MainVPC"
}
}
This configuration defines a VPC with DNS support enabled.
Step 3: Initialize Terraform
Before applying any changes, initialize Terraform in the project directory:
terraform init
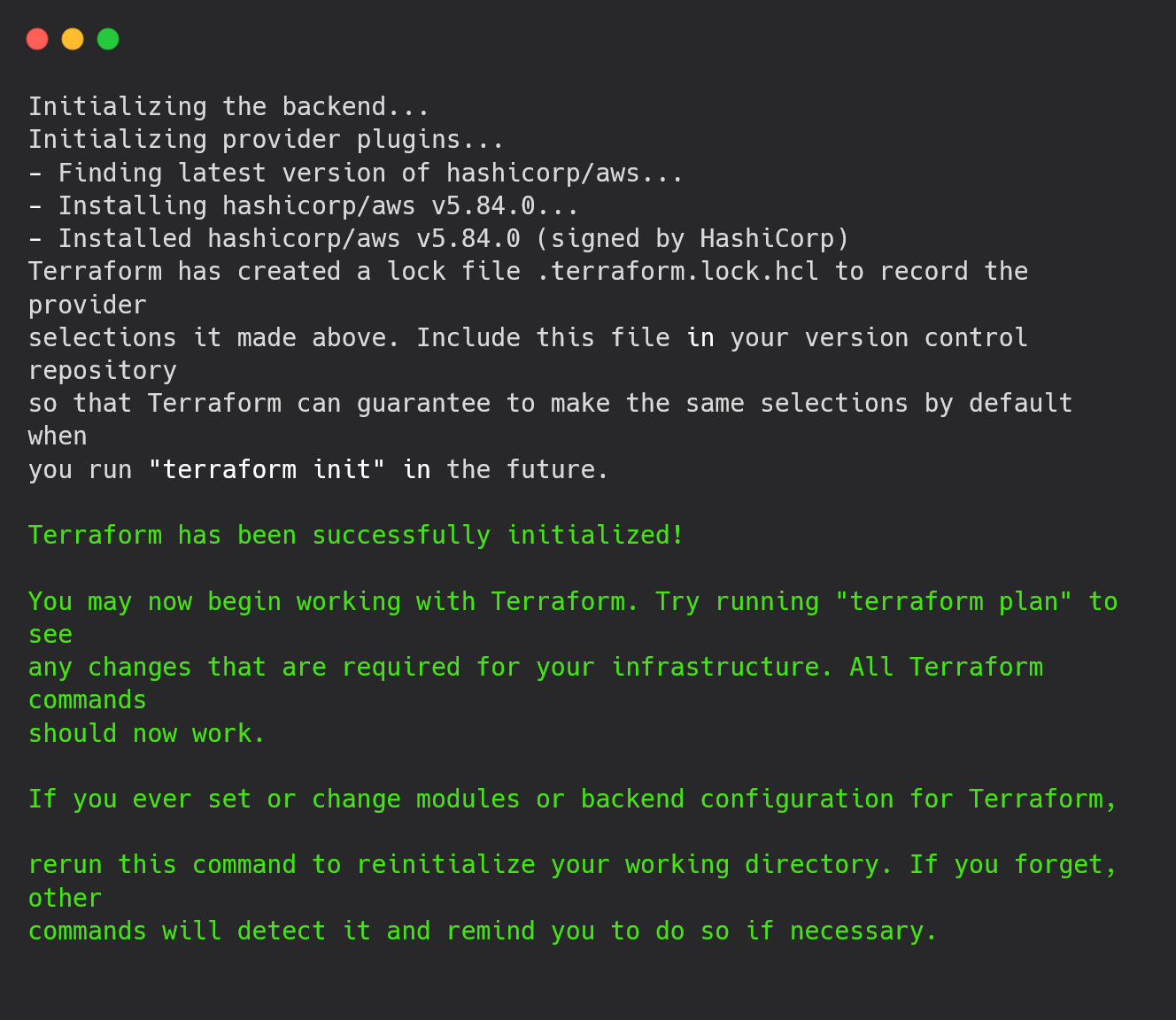
This downloads the necessary provider plugins and prepares Terraform for execution.
Step 4: Stage and Commit Changes
Once the Terraform files are ready, they should be added to Git:
git add vpc.tf
git commit -m "Added VPC configuration"

This makes sure that the changes are saved in the feature
branch.
Step 5: Push the Feature Branch to Remote Repository
To share the changes with the team, push the feature branch to the remote repository:
git push origin feature/add-vpc
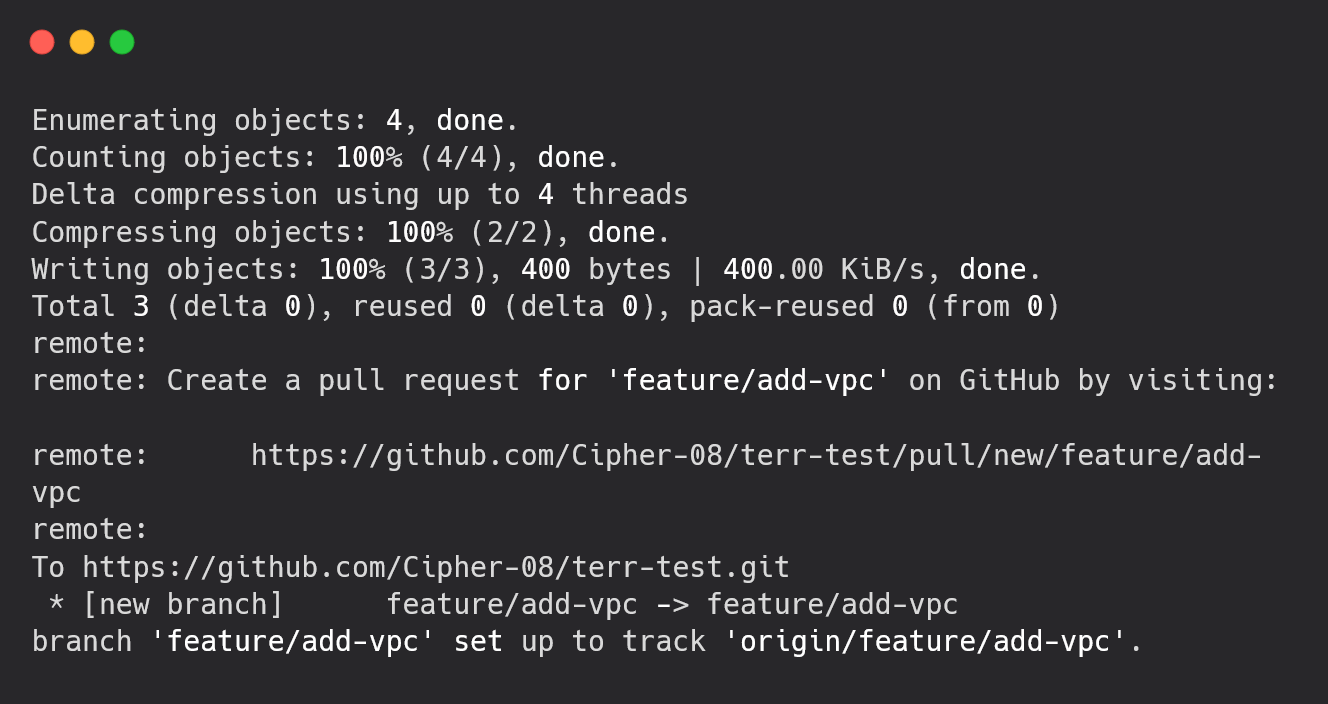
Now, the branch is available in the remote repository for collaboration and review.
Validating and Testing Terraform Changes
Before merging, the configuration must be tested to ensure it works as expected. Terraform provides commands to validate and preview changes before applying them.
Step 1: Validate Terraform Configuration
Run the following command to check for syntax errors:
terraform validate

If there are any issues, Terraform will display error messages that need to be fixed before proceeding.
Step 2: Preview Changes with Terraform Plan
To see what Terraform will create, run:
terraform plan
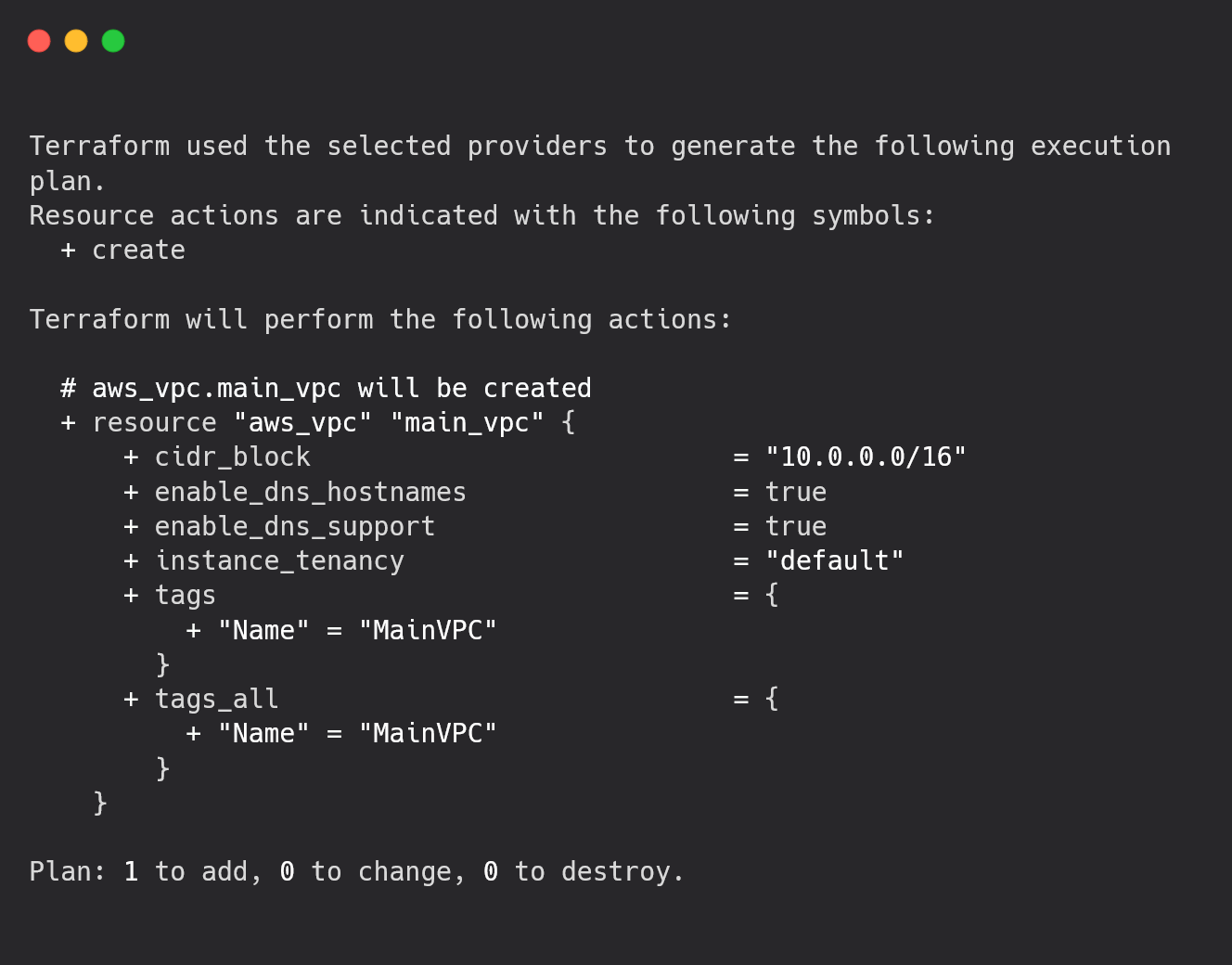
Terraform will show a list of resources it intends to create. If everything looks correct, we can move to the next step.
Step 3: Apply Terraform Changes
To create the VPC in AWS, execute:
terraform apply -auto-approve
This command deploys the VPC as defined in vpc.tf. The -auto-approve
flag skips the confirmation prompt.
Once the process is completed, Terraform will display the created resources.
Finishing the Feature Branch
After testing the changes, the feature
branch can be merged back into develop
to make the update available for further testing and deployment.
Step 1: Merge the Feature Branch
First, switch back to the develop
branch:
git checkout develop

Then, merge the feature branch:
git flow feature finish add-vpc
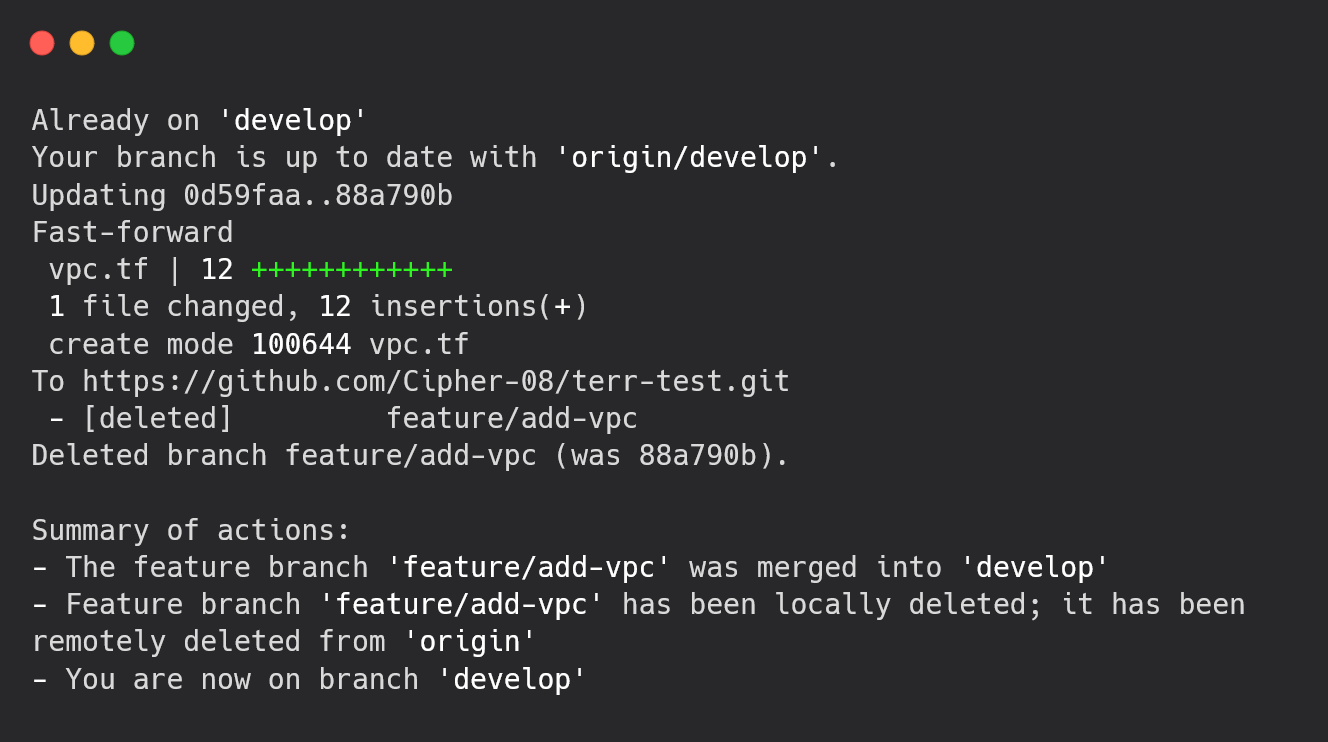
This command merges feature/add-vpc
into develop
and deletes the feature
branch locally.
Step 2: Push Changes to Remote Repository
To make sure that the updated develop
branch is available for the team, push the changes:
git push origin develop
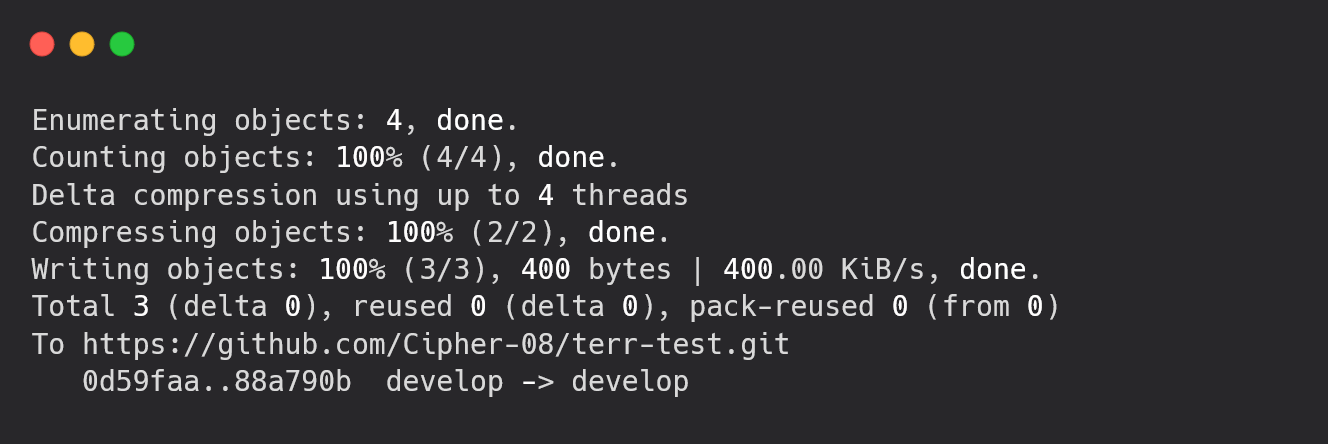
Now, the VPC configuration is stored in develop
, ready for further testing or inclusion in a release.
Managing Releases and Hotfixes
Once infrastructure changes have been merged into develop
, the next step is to manage releases and handle any urgent fixes. GitFlow provides a structured way to prepare updates for production and apply urgent changes without disrupting ongoing development.
Preparing a Release Branch
A release branch is created when infrastructure changes are ready to be deployed. This allows final testing and adjustments before merging into main
.
Step 1: Create a Release Branch
To start a new release, run:
git flow release start v1.0
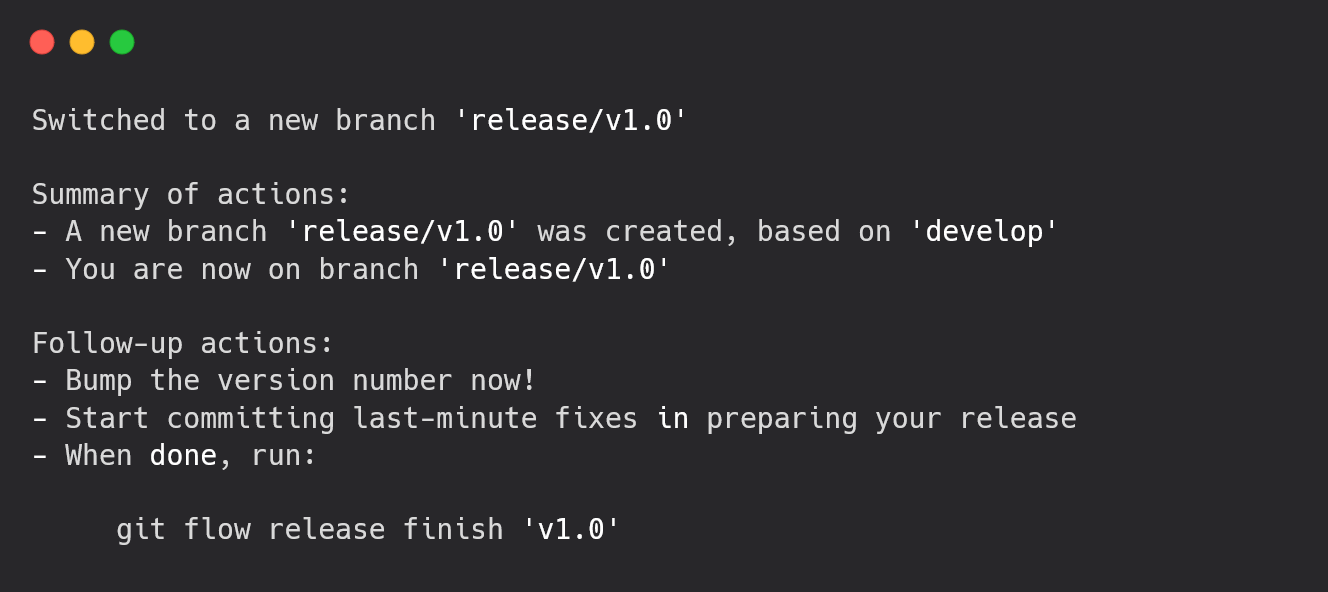
This creates a release/v1.0
branch from develop
, allowing teams to finalize configurations.
Step 2: Verify Infrastructure Changes
Before deployment, run Terraform in a test environment to confirm that everything works as expected:
terraform plan
terraform apply
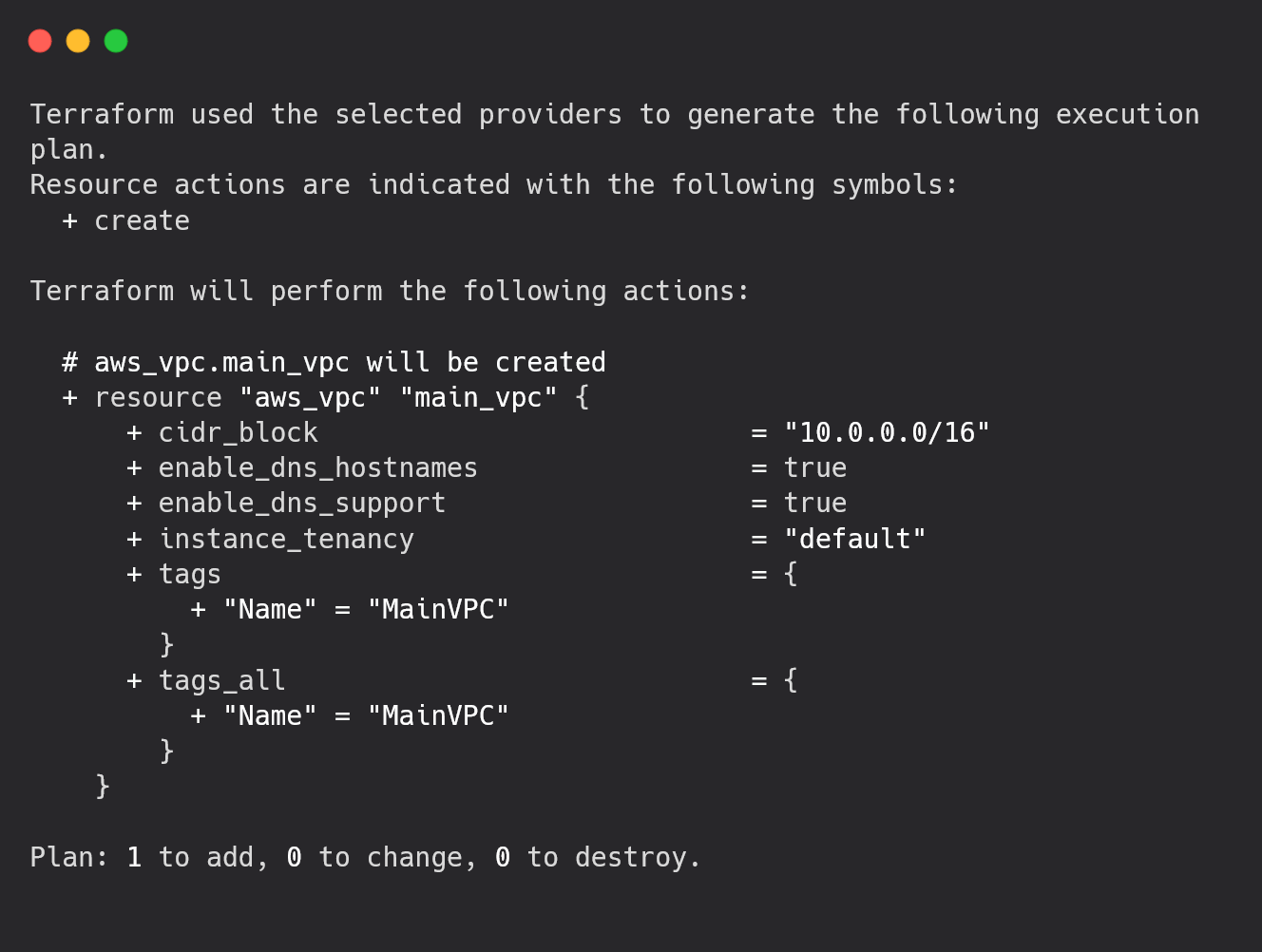
If any issues are found, fixes should be applied in the release
branch before proceeding.
Step 3: Tag and Merge the Release
Once testing is complete, tag the release and merge it into main
:
git flow release finish v1.0
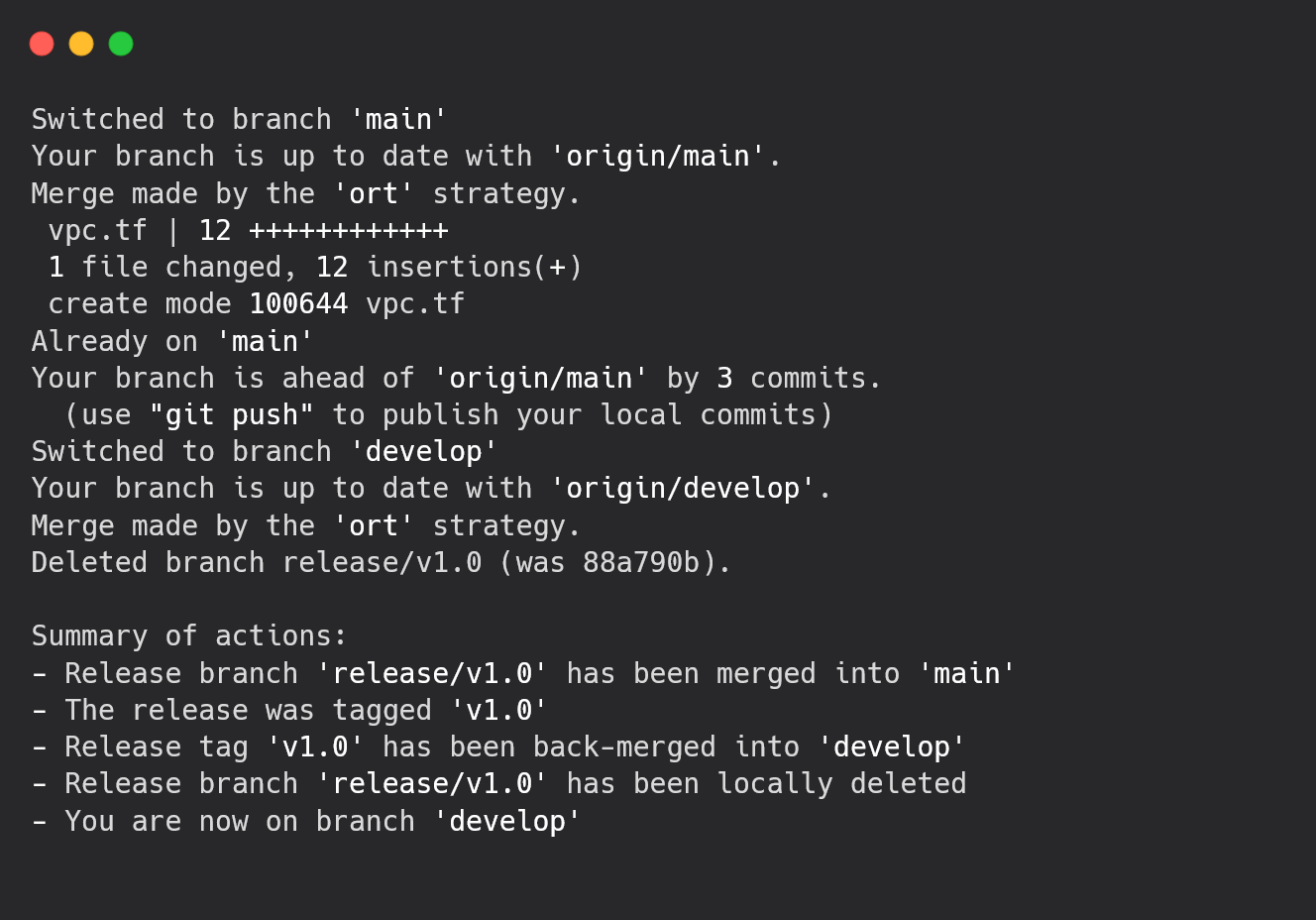
This command:
- Merges
release/v1.0
intomain
- Tags the
release
version - Merges changes back into
develop
- Deletes the
release/v1.0
branch locally
Finally, push the updates to the remote repository:
git push origin main --tags
git push origin develop
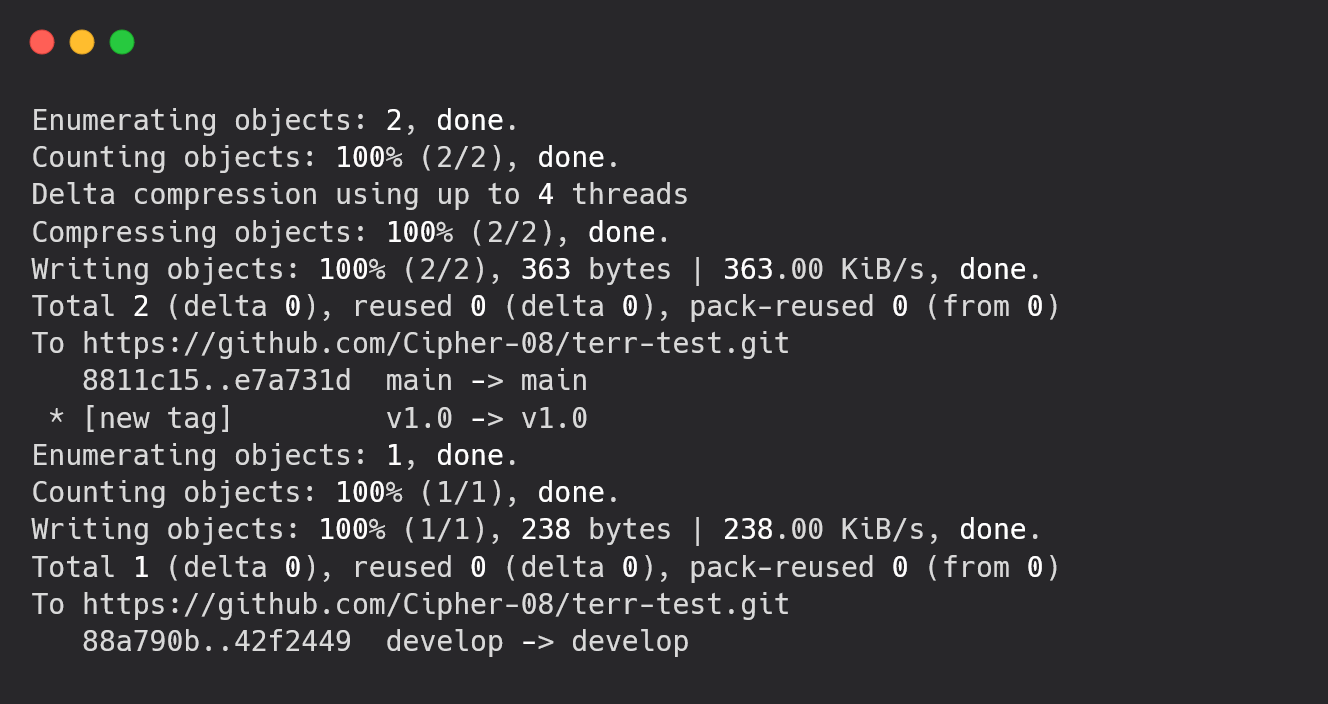
At this point, the infrastructure changes are in main
and ready for production deployment.
Applying Hotfixes in Production
Hotfixes are used to quickly fix production issues, such as security misconfigurations or failures, without affecting ongoing development.
Step 1: Create a Hotfix Branch
If an urgent fix is needed, create a hotfix branch from main
:
git flow hotfix start fix-iam-policy
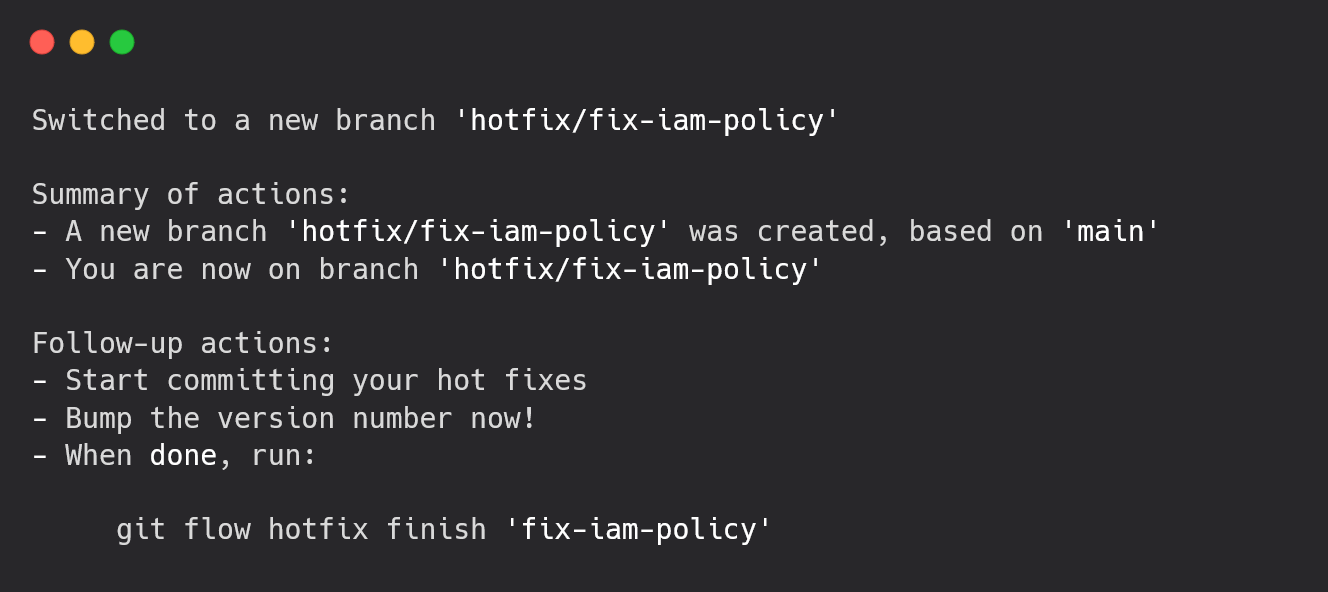
This makes sure that the fix is isolated from other development work.
Step 2: Apply the Fix and Test
Make the necessary changes to the Terraform configuration. For example, if an IAM policy needs to be corrected, update the relevant Terraform files, then test the changes:
git flow hotfix finish fix-iam-policy
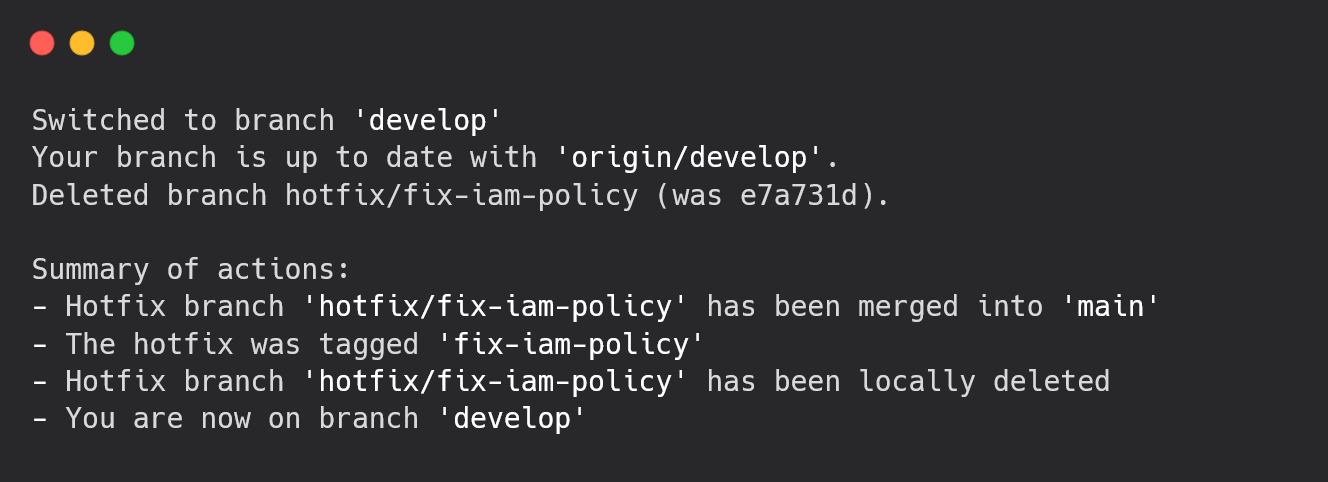
This command:
- Merges
hotfix/fix-iam-policy
intomain
- Tags the fix
- Merges it back into
develop
- Deletes the
hotfix/fix-iam-policy
branch
Finally, push the changes to the remote repository:
git push origin main --tags
git push origin develop
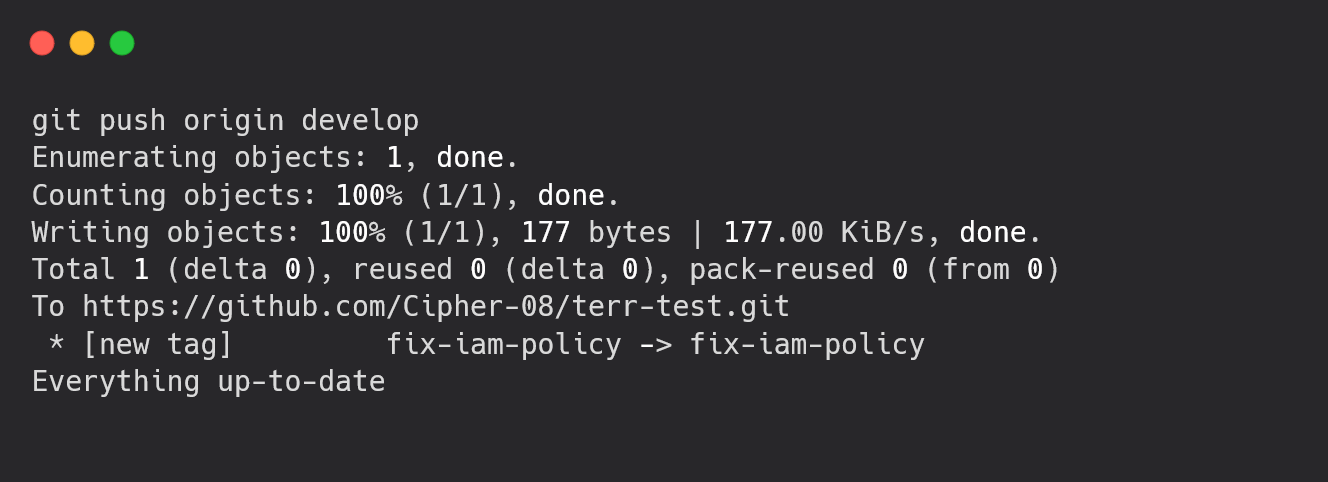
The fix is now applied to production while keeping the development environment in sync.
Now managing Terraform with GitFlow workflow without any automation can be a bit difficult. Every infrastructure change has to follow a strict branching model, and Terraform should only run at the right stages. But without an automated system, it’s easy for mistakes to happen. Changes might get applied too early, updates might go untested, or an important fix might take longer than it should. Teams had to enforce these rules manually.
A feature branch would be created for every infrastructure update. After testing, the branch would merge into develop
, and when a release was ready, develop
would merge into release/*
. Terraform changes had to be applied at this stage to keep the environment stable. If something broke in production, a hotfix/*
branch had to be created, merged into main
, and then merged back into develop
to maintain consistency.
Every step needed careful review to make sure that Terraform wasn’t applied at the wrong time. If someone ran terraform apply
in a feature
branch or merged into the wrong branch, it could lead to unexpected changes or drift in infrastructure.
Here comes Terrateam. Instead of relying on manual enforcement.
GitFlow Workflows with Terrateam
Terrateam automates Terraform workflows based on GitFlow. It makes sure Terraform only runs when changes reach the right branch. By defining destination branches in .terrateam/config.yml, Terraform can be triggered automatically at the correct stage.
To enforce GitFlow with Terrateam, update the **.terrateam/config.yml **file:
destination_branches:
- branch: "release/*"
- branch: "main"
source_branches: ["hotfix/*"]
This configuration makes sure that:
- Terraform operations run only when merging
develop
intorelease/*
orrelease/*
intomain
. - Hotfix branches (
hotfix/*
) are allowed to merge intomain
for urgent fixes.
With this setup, Terrateam automatically applies Terraform changes only when they reach the correct branches, preventing accidental deployments from feature
or development
branches.
Once a pull request is raised, Terrateam automatically runs terraform plan
, providing a detailed preview of the changes that will be applied. After reviewing the plan, the next step is to apply the changes. To proceed, a team member needs to comment with terrateam apply
.
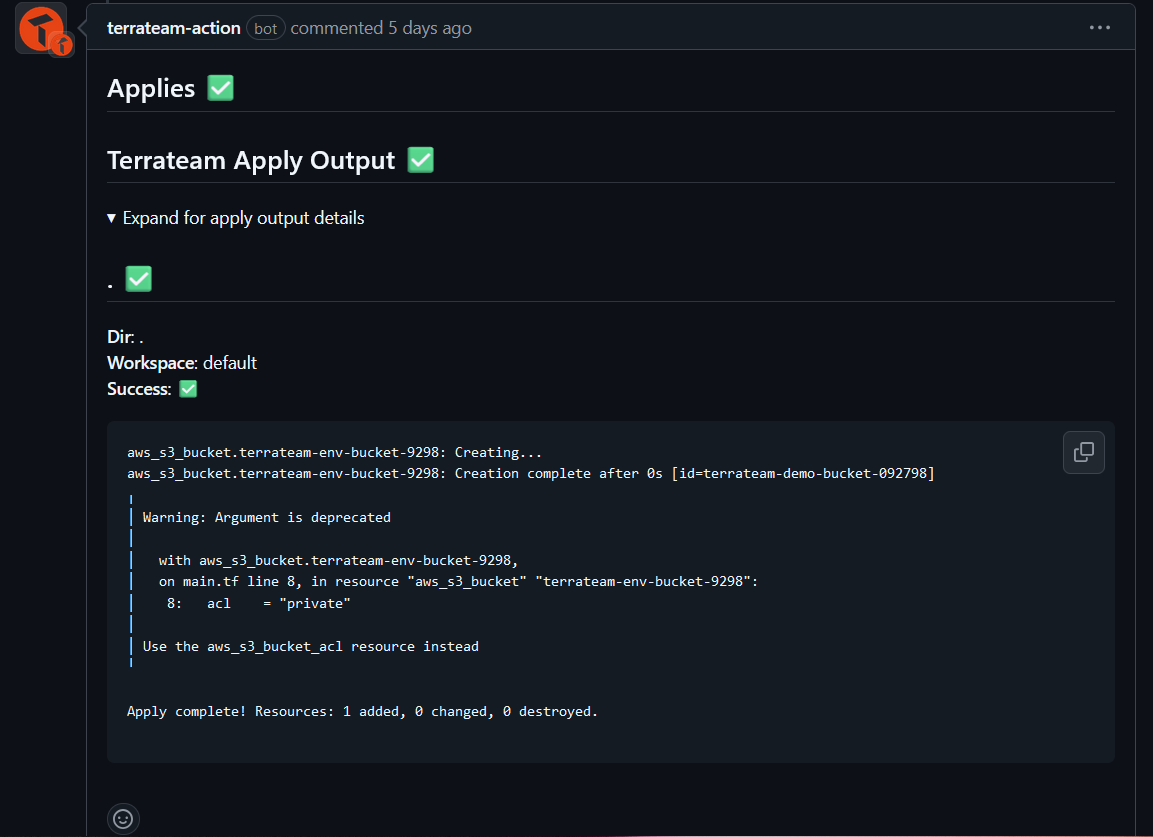
By integrating Terrateam with GitFlow, Terraform workflows become more reliable. There’s no risk of running changes too early or applying updates in the wrong order. Teams can focus on making changes, knowing that deployments will always happen at the right time, in the right way.
Conclusion
Till now, you should have a clear understanding of how GitFlow helps manage Terraform changes in a structured way. By following this workflow, teams can keep infrastructure updates organized, prevent conflicts, and ensure changes are tested before deployment. This approach makes infrastructure management more predictable and easier to maintain.
Frequently Asked Questions
Q. What is GitFlow used for?
GitFlow is a branching model that helps teams manage code changes in a structured way, ensuring that development, releases, and hotfixes follow a clear workflow.
Q. What is the difference between GitHub workflow and GitFlow?
GitHub workflow focuses on pull requests and CI/CD automation, while GitFlow defines a structured branching model for managing feature development, releases, and hotfixes.
Q. What are feature branches?
Feature branches are temporary branches created from develop
to work on specific updates or new functionality before merging them back after testing.
Q. What are the best practices for GitFlow?
Use separate branches for features, releases, and hotfixes, always merge feature branches into develop
, and ensure releases go through release/*
before merging into main.