Terraform Best Practices For CI/CD Pipelines
Terraform allows organizations to automate the deployment and configuration of cloud and on-premises resources, ensuring consistency and reliability. With Terraform, infrastructure becomes predictable and version-controlled, helping teams manage multi-environment setups efficiently. By using infrastructure as code, teams can define Terraform configurations once and reuse those configurations across multiple environments, ensuring consistency within your infrastructure. Terraform commands like terraform init
, terraform validate
, and terraform plan
simplify the Terraform workflow by automating setup, validating configurations, and previewing changes before deploying them.
Using Terraform in CI/CD pipelines, however, brings a lot of challenges. Managing remote backends and securing environment variables becomes more difficult as infrastructure scales. Configuration drift, caused by changes done through CLI or cloud console, can result in mismatches between the planned and actual infrastructure state. DevOps teams sometimes skip testing Terraform configuration, increasing the risk of errors during the deployment of resources. Debugging pipeline failures and implementing role-based access control also add a bit of complexity to the overall task.
To address these issues, storing state files in a remote backend like AWS S3 or Azure Blob Storage ensures secure and centralized access. Role-based access control makes sure that only authorized team members can make changes to your infrastructure.
These practices improve consistency, reduce deployment errors, and simplify your infrastructure management. This blog will explore actionable strategies for securing CI/CD pipelines, managing remote backends, and optimizing Terraform workflows.
How Can You Apply Terraform Best Practices in Your CI/CD Workflow?
Let’s say you are a DevOps engineer at an organization. You apply a Terraform configuration in your development environment, and it works perfectly. However, when the same configuration runs in the CI/CD pipeline, it fails. This might be due to mismatched configurations between both environments, missing environment variables, or inconsistencies between local and CI/CD pipeline setups. Debugging such issues can consume hours, delay deployments, and risk errors in your production environment.
How to Scale Infrastructure Using Modular Terraform Code
Modularity in Terraform means organizing your code into reusable components, also known as modules. Each module is designed to perform a specific task, like creating an EC2 instance, setting up an S3 bucket, or configuring a database. A module in Terraform combines related resources, such as security groups, IAM roles, and instance configurations, into one. This makes the code easier to manage and reuse. For example, you can use the same module to set up a new environment or onboard a new client without rewriting the Terraform configuration.
To create a reusable Terraform module, define resources like an S3 bucket in a separate directory. Then, reference the module in your main configuration with the module
block, passing required variables like bucket_name
and tags
. This enables code reuse, scalability, and cleaner infrastructure.
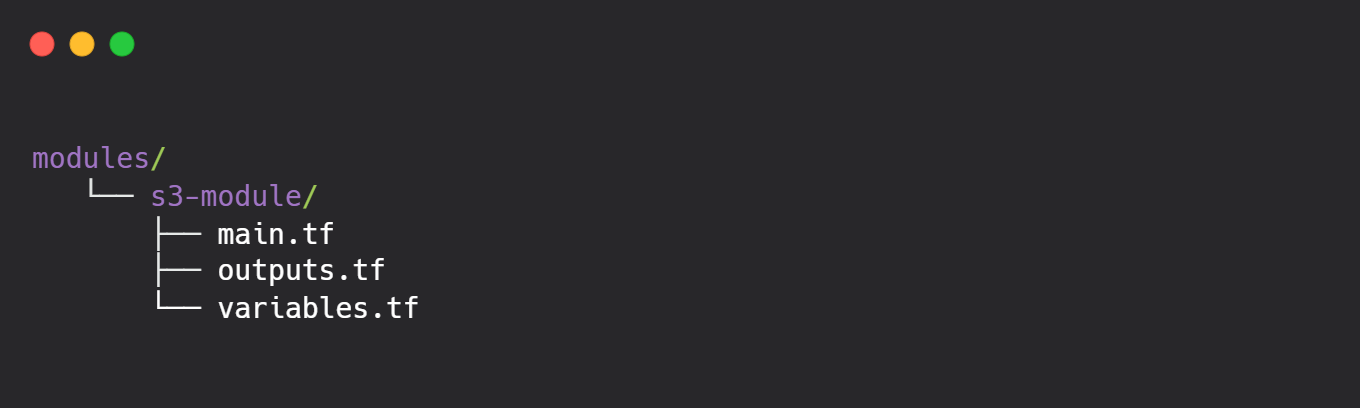
Each configuration file serves a specific purpose to separate configuration, inputs, and outputs. This directory is a Terraform module (s3-module
) with:
main.tf
: Defines S3-related resources.variables.tf
: Contains input variables for customization.outputs.tf
: Exposes key outputs like bucket name or ARN.
Modularizing your code prepares your infrastructure for scaling while keeping it maintainable and efficient at the same time.
Organizing Terraform Directory Structure
Efficient infrastructure management begins with a clear directory structure for your Terraform configurations. This involves separating Terraform code for environments like development, staging, and production while keeping configurations for different clients or teams organized. Without a well-structured setup, managing environment variables, validating Terraform configurations, and running terraform plan
or terraform apply
becomes a bit difficult, leading to misconfigurations and deployment delays.
Key Features of an Effective Terraform Directory Structure
- Client-Specific Directories:
Each client should have a dedicated folder to keep their configurations separate. Inside this folder, create subdirectories for each environment dev/
, staging/
, and prod/
to manage environment-specific settings. This approach keeps configurations isolated, making it easier to apply changes to one client or environment without affecting others.
- Global Configurations:
The global/
directory should store configuration files that apply across all environments for each client. This includes provider settings, backend configurations, and shared variables. By centralizing these configurations, you ensure consistent behavior across environments and simplify management when changes are needed, such as switching providers or modifying backend settings.
This structure ensures your Terraform workflows remain organized, efficient, and scalable.
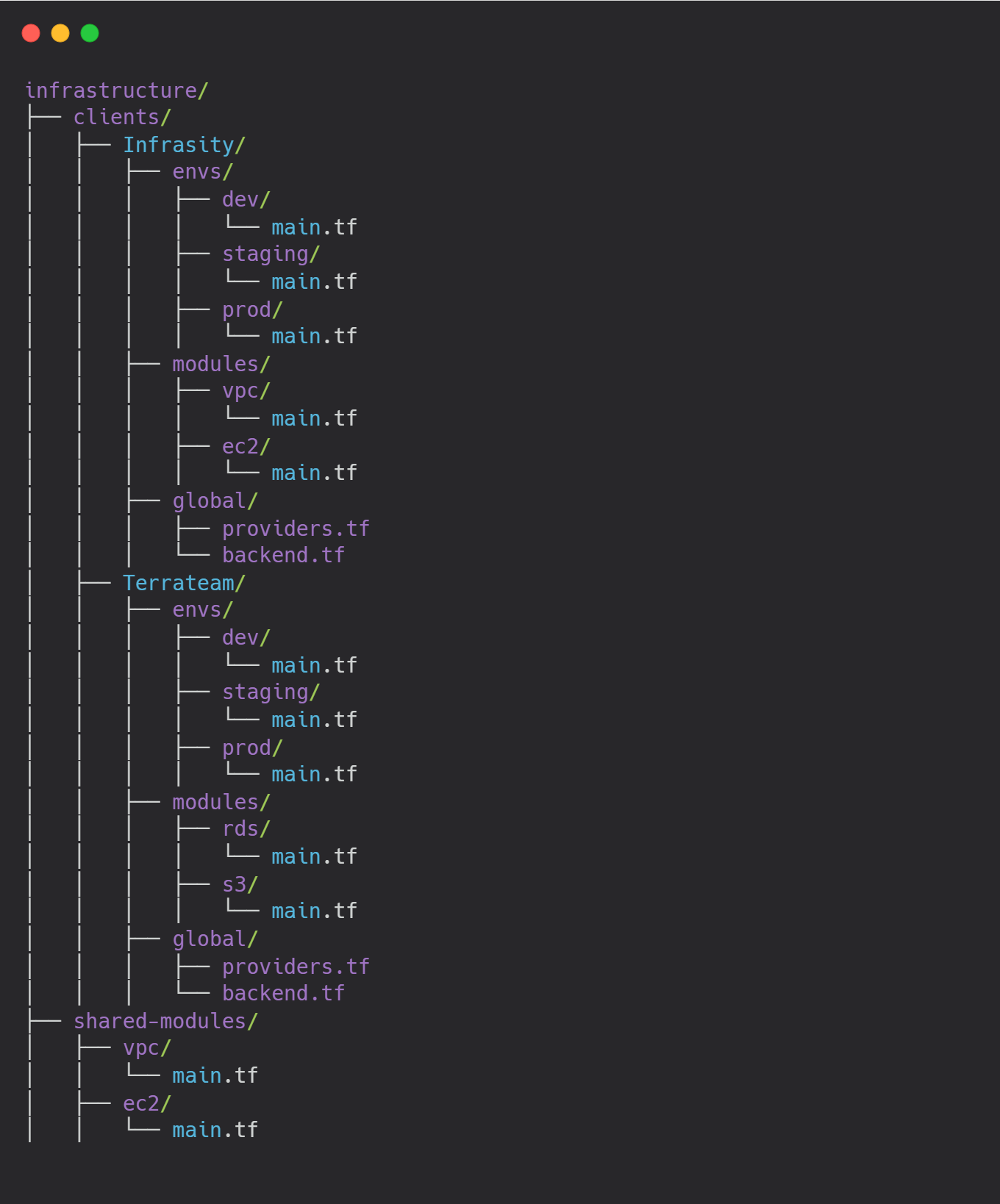
This is a Terraform directory structure for managing infrastructure:
clients/
: Contains configurations for multiple clients (e.g., Infrasity, Terrateam).envs/
: Separate environments (dev
,staging
,prod
) for each client.modules/
: Client-specific modules (e.g.,vpc
,ec2
,rds
,s3
).global/
: Shared client-wide configurations (e.g.,providers.tf
,backend.tf
).
shared-modules/
: Centralized reusable modules (e.g.,vpc
,ec2
) shared across clients.
This structure supports modularity, multi-client setups, and environment isolation.
A well-structured directory is important for managing infrastructure efficiently, especially with multiple environments or clients. Separating configurations, using reusable modules, and isolating environments minimize errors, enhance collaboration, and ensure scalability. A clear structure keeps Terraform workflows organized and manageable.
To avoid these issues and ensure reliable Terraform deployments within your CI/CD pipeline, here are some best practices and actionable steps that should be followed when working with Terraform within CI/CD pipelines.
Best Practices for Securing Secrets in a CI/CD Pipeline
In infrastructure management, secrets like API keys, database credentials, and cloud provider tokens are critical for secure communication between services. However, hardcoding them in Terraform files or exposing them in CI/CD pipelines can lead to serious security risks, including unauthorized access and data breaches. To avoid this, secrets should always be managed securely, ensuring they are never exposed in code or logs during deployment.
-
Storing secrets directly in Terraform files or version control systems like Git.
-
Using unencrypted environment variables in CI/CD pipelines, making them accessible to anyone with access to the pipeline.
-
Failing to centralize the management of sensitive information leads to inconsistent and insecure storage practices.
Proper handling of secrets is essential to ensure they remain protected throughout your infrastructure lifecycle.
Removing Hardcoded Secrets from Terraform Configurations
Hardcoding secrets directly in Terraform configurations is a risky practice that exposes sensitive information in code. This makes it easier for secrets to be accidentally leaked or accessed by unauthorized parties. Here's how to properly manage secrets in Terraform without hardcoding them.
Managing Secrets with CI/CD Built-In Tools
Most CI/CD platforms, like GitHub Actions and Azure DevOps, etc, offer built-in secret management tools to store sensitive information like API keys and tokens securely. These features prevent secrets from being exposed in logs or code repositories. Here’s a guide on managing secrets in GitHub Actions.
Storing Secrets in GitHub Actions
To securely manage secrets in GitHub Actions, follow these steps to store sensitive information like API keys or database credentials:
-
Access your repository settings: Go to your GitHub repository and click on the Settings tab.
-
Navigate to Secrets: In the left sidebar, under the Secrets and Variables section, click on Actions.
-
Create a new secret: Click on the New Repository secret button.
-
Define the secret: In the "Name" field, enter a clear name for your secret, such as
DB_PASSWORD
orAWS_ACCESS_KEY
. In the"Value"
field, input the sensitive value (e.g., your actual database password or API key). -
Save the secret: Click on the Save secret button to securely store it.
GitHub will encrypt the secret and store it in your repository’s settings. It will be accessible during workflow runs, ensuring the secret remains hidden from code, logs, or anyone else accessing the repository. This method ensures that sensitive information is protected and not exposed in your Terraform configurations or CI/CD pipeline.
You can reference stored secrets in your workflow file using the secrets context. For example, to pass a database password as an environment variable:
name: Deploy Infrastructure
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Check Out Code
uses: actions/checkout@v3
- name: Use Secret in Terraform
env:
DB_PASSWORD: ${{ secrets.DB_PASSWORD }}
run: |
echo "Setting up infrastructure."
terraform apply -var="db_password=$DB_PASSWORD"
This GitHub Actions workflow is triggered on a push to the main branch. It defines a deployment job that runs on an Ubuntu machine. The workflow includes two key steps: first, it checks out the repository code using the actions/checkout action to ensure the latest code is available for the deployment process. Second, it securely accesses the DB_PASSWORD
secret from GitHub's secret store, exposes it as an environment variable, and uses it in the terraform apply command to pass the db_password
variable. This setup ensures a secure and automated infrastructure deployment process whenever changes are pushed to the main branch.
By using GitHub Actions' built-in secret management, you avoid hardcoding sensitive information in your workflows or code. This method keeps your secrets secure while enabling smooth, automated deployments.
Use Remote Backends for State Management
Terraform uses a state file, terraform.tfstate, to track your infrastructure’s current status and identify changes to apply. By default, this file is stored locally, which can cause conflicts or lost updates when multiple team members work on the same infrastructure. Remote backends, like Amazon S3, Azure Blob Storage, or HashiCorp Consul, store the state file in a centralized, secure location. This ensures all team members access the same, up-to-date state, enabling smoother collaboration and consistent deployments.
Why Should You Use Remote Backends in Terraform?
When managing infrastructure with Terraform, using remote backends offers several key advantages that improve team collaboration, deployment consistency, and security.
- Team Collaboration:
For small teams or solo developers, a local state file may work. In larger teams, a remote backend is crucial to ensure everyone shares a single state file. This prevents inconsistencies, conflicting changes, and overwrites, making collaboration smoother and reducing errors.
- Consistent Deployments:
A remote backend centralizes the state file, ensuring that all infrastructure changes are tracked in one place. This prevents discrepancies between local state files and the actual deployed infrastructure. With a centralized state, every deployment reflects the most up-to-date state of your infrastructure, making it easier to manage and avoid unexpected issues.
- Improved Security:
State files often include sensitive data like resource IDs, passwords, or secret outputs. Storing them locally risks unauthorized access or accidental leaks. Remote backends, such as AWS S3 or Azure Blob Storage, offer secure, centralized storage with encryption and access controls, ensuring sensitive data stays protected.
Enabling State Locking
State locking ensures that only one Terraform operation, e.g., terraform plan
and terraform apply
, can modify the state file at a time. Without locking, simultaneous operations could corrupt the state file, leading to unpredictable infrastructure changes.
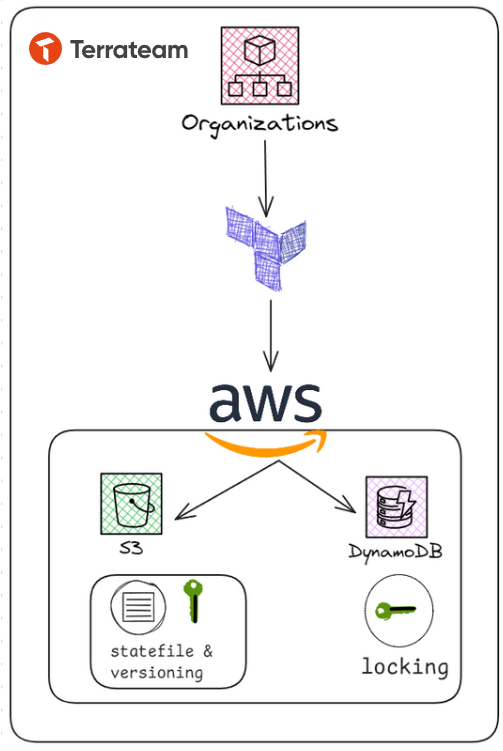
Configure the Backend in Terraform:
To configure a remote backend in Terraform, follow these steps:
-
Define the backend: In your backend.tf file, specify the backend provider (e.g., AWS S3, Azure Blob Storage). Example for AWS S3.
-
Initialize the backend: Run terraform init to set up the remote backend and migrate the state file.
-
Verify the setup: Terraform will now use the remote backend for future operations, ensuring centralized state management.
terraform {
backend "s3" {
bucket = "terraform-state-bucket"
key = "envs/prod/terraform.tfstate"
region = "us-west-2"
dynamodb_table = "terraform-lock-table"
}
}
Breakdown of the Code:
-
Bucket: Specifies the name of the S3 bucket (terraform-state-bucket) where Terraform will store the state file. The bucket must already exist in AWS.
-
Key: Sets the path to the state file within the S3 bucket. In this example, the state file is stored under the
envs/prod/
directory with the filename terraform.tfstate. This helps organize the state files by environment, making it easier to manage different stages like development, staging, and production. -
Region: Defines the AWS region where the S3 bucket is located (in this case, us-west-2).
-
Dynamodb_table: Specifies the DynamoDB table (terraform-lock-table) used for state locking. This prevents multiple users from applying changes to the state file simultaneously, ensuring that the state remains consistent and avoiding potential corruption.
This setup ensures secure, consistent, and collaborative management of your Terraform state.
What Makes Remote Backends Important?
Using remote backends in Terraform offers several significant advantages that improve collaboration, security, and the reliability of your infrastructure deployments. Below are the key reasons why remote backends are essential:
-
Conflict Prevention State locking ensures that only one user or process can modify the state file simultaneously, preventing conflicts and errors in a collaborative environment.
-
Centralized Management A remote backend stores the state file in a single, central location, ensuring all team members access the same version and reducing the risk of state drift.
-
Increased Security Remote backends like AWS S3 offer encryption and fine-grained access controls (e.g., IAM policies), keeping sensitive data safe and restricting unauthorized access.
-
Versioning of State file Remote backends handle large, complex infrastructure projects, making it easy for distributed teams to access and update the state file consistently and securely.
Remote backends enable secure, centralized state management, preventing conflicts with state locking and protecting sensitive data. They ensure consistent deployments and streamline collaboration for teams in enterprise environments.
Using Tags
Tags are key-value pairs assigned to resources that help you organize, identify, and manage them more effectively. Tagging resources enhances visibility and control across your infrastructure, making it easier to track usage, allocate costs, and implement governance policies.
Consistently applying tags across all Terraform-managed resources ensures:
-
Improved Organization: Resources are grouped in a meaningful way, making it easier to manage large-scale infrastructure.
-
Efficient Cost Allocation: By tagging resources with cost-related labels, you can allocate costs more accurately across teams, projects, or environments.
-
Better Governance: Tags help enforce policies for resource management, enabling easier auditing, compliance, and reporting.
-
Faster Troubleshooting: With well-defined tags, identifying and resolving issues becomes quicker by filtering resources based on their attributes (e.g., environment or application).
By integrating tags into your Terraform configurations, you make resource management more transparent, efficient, and scalable, improving both day-to-day operations and long-term infrastructure governance.
For example, you can use tags to categorize resources by environment (e.g., dev, prod), application (e.g., web-server, database), owner (e.g., team1, team2), or cost center (e.g., marketing, engineering). This allows you to filter, search, and manage resources based on these categories.
resource "aws_instance" "devlop" {
ami = "ami-0e2c8caa4b6378d8c"
instance_type = "t2.micro"
tags = {
Enviroment = "Dev"
Project = "App1"
Owner = "TeamA"
}
}
-
Environment: Indicates the stage or environment the resource is used in, such as Dev, Staging, or Prod. This helps separate resources by their purpose and lifecycle.
-
Project: Identifies the specific project or application the resource belongs to, such as WebApp or Database. This makes it easier to group resources by their related tasks.
-
Owner: Specifies the team or person responsible for the resource, such as DevOps or Team1. This helps with accountability and resource management.
In conclusion, tagging resources in Terraform is a crucial practice for enhancing visibility, managing costs, and ensuring proper governance. By consistently applying tags and following a structured tagging policy, you can streamline resource management, improve organization, and maintain compliance across your entire infrastructure.
Implementing Security with Checkov
Consider an organization using Terraform to deploy its cloud infrastructure, including an S3 bucket to store sensitive customer data. A DevOps engineer, in the rush of deployment, inadvertently omits the encryption setting for the S3 bucket. This oversight goes unnoticed until weeks later, when the bucket is accessed by an unauthorized party, exposing sensitive data and leading to a costly data breach.
This scenario highlights the importance of enforcing security best practices during infrastructure deployment. By using tools like Checkov, organizations can automatically scan Terraform configurations for security misconfigurations, such as missing encryption or insecure access controls, before they are deployed, significantly reducing the risk of such vulnerabilities making it to production.
Without Checkov:
Without tools like Checkov, securing infrastructure requires manual efforts like writing scripts to find issues (e.g., open security groups or missing encryption) or checking configurations via the cloud provider’s GUI or CLI. These methods are slow, error-prone, and hard to scale as infrastructure grows. This section explains the challenges of managing Terraform security manually and the importance of using tools like Checkov to simplify the process.
-
Encryption configurations:
Ensuring encryption is enabled on cloud resources is critical to protect sensitive data. For example, S3 buckets, databases, and EBS volumes often store confidential information, and without proper encryption, this data can be vulnerable to unauthorized access. During a manual review, it’s essential to check that encryption settings are explicitly defined in the resource configurations.
resource "aws_s3_bucket" "my_bucket" {
bucket = "secure-bucket"
server_side_encryption_configuration {
rule {
apply_server_side_encryption_by_default{
sse_algorith = "AES256"
}
}
}
}
If encryption is missing or incorrectly configured, sensitive data could be exposed, leaving the system vulnerable to breaches.
-
IAM Policies:
IAM policies manage who can access your cloud resources and what they can do. Overly permissive policies, such as using wildcard (*) permissions, create security risks by granting unrestricted access, violating the principle of least privilege. Security reviews should replace such policies with restrictive ones that allow only necessary actions and access.
Grants full access to all actions and resources, which is not recommended as it provides administrative-level access to the user. This can lead to significant security risks, especially when not using tools like Checkov to find and fix such issues.
{
"Effect": "Allow",
"Action": "*",
"Resource": "*"
}
Allows only s3:PutObject and s3:GetObject actions on a specific S3 bucket, enabling upload and retrieval of objects while restricting other actions to enhance security.
{
"Effect": "Allow",
"Action": ["s3:PutObject", "s3:GetObject"],
"Resource": "arn:aws:s3:::my-secure-bucket/*"
}
By focusing on encryption and refining IAM policies, you can significantly improve the security posture of your cloud infrastructure. Properly securing data and access controls helps prevent potential vulnerabilities and ensures compliance with best security practices.
Challenges of Manual Reviews
-
Time-Consuming: Reviewing infrastructure manually requires inspecting each file and configuration individually, which becomes inefficient as the project grows. For large infrastructures with hundreds of resources, this process can take a considerable amount of time.
-
Error-Prone: Manual reviews are subject to human error. It’s easy to overlook critical settings or configurations in complex environments, leading to missed security risks or misconfigurations.
-
Difficult to Scale: As infrastructure expands, manually reviewing every resource becomes increasingly unmanageable. This makes it hard to keep up with security audits, especially in fast-paced or large-scale environments.
Without automated tools like Checkov, manual reviews rely heavily on continuous effort and expertise, making it challenging to identify and fix security issues effectively.
With Checkov:
Checkov simplifies infrastructure security by automatically detecting misconfigurations in Terraform code and enforcing security policies. It replaces manual reviews with automated scans against predefined and custom rules, ensuring consistent, accurate checks and saving time.
How Checkov Works
Checkov automates the detection of misconfigurations in Terraform code to improve security. It scans for critical issues such as:
-
Unencrypted Storage: Identifies resources like S3 buckets or EBS volumes that lack encryption, ensuring sensitive data is properly protected.
-
Open Security Groups: Flags security groups with rules allowing unrestricted access (e.g.,
0.0.0.0/0
), helping prevent publicly exposing resources. -
Overly Permissive IAM Roles: Detects IAM policies with wildcard (*) permissions, violating the least privilege principle and increasing the risk of unauthorized access.
By automating these checks, Checkov ensures security gaps are caught early, reducing the risk of misconfigurations in your infrastructure.
-
Enforces Security Policies
Checkov automatically enforces security policies by scanning Terraform code against predefined or custom rules. For instance, it ensures all resources are properly tagged (e.g., Environment, Owner) and verifies that databases are deployed in private subnets for better security. You can also create custom policies in YAML or Python to meet your organization’s specific needs.
Integrating Checkov into CI/CD Pipelines
To automate security checks, integrate Checkov into your CI/CD pipeline. For example, in GitHub Actions, you can add a step to scan Terraform code during the build process. This ensures security issues are caught early, preventing insecure deployments and maintaining consistent standards across your infrastructure.
jobs:
checkov:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: action/checkout@v2
- name: Run Checkov
run: checkov -d ./terraform
To integrate Checkov into a GitHub Actions CI/CD pipeline, define a job (e.g., checkov) in the workflow file and set runs-on: ubuntu-latest for the runner. Add steps to clone the repository using actions/checkout@v2
and scan Terraform code with checkov -d ./terraform
. This automates security checks, prevents insecure deployments, and ensures compliance in every pipeline execution.
The output for the above code is like this :
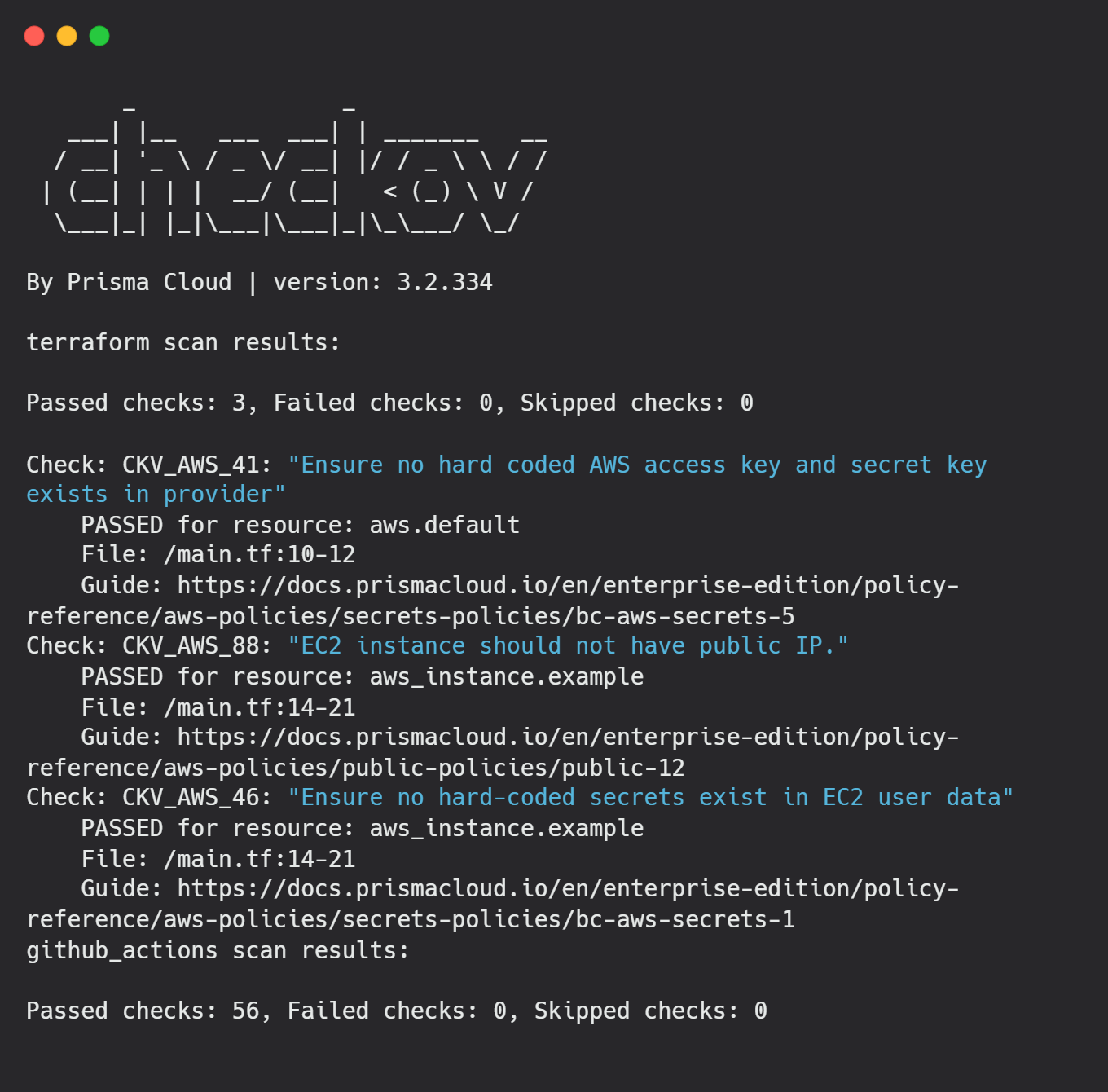
Checkov makes securing infrastructure faster, easier, and more reliable by automating misconfiguration detection and policy enforcement. It replaces the need for manual reviews and custom scripts, ensuring consistent compliance with security best practices.
Importing Existing Infrastructure
Managing infrastructure manually through the cloud console makes it difficult to track changes, maintain consistency, and debug issues as the setup grows. Without version control, it’s hard to see everything deployed or scaled effectively. Moving to Terraform lets you automate deployments, track all changes in code, and ensure a consistent and manageable setup.
Here’s how to use terraform import
step by step to sync your existing infrastructure with Terraform.
Steps to Import Existing Infrastructure
- Create the Terraform Configuration:
Write a Terraform configuration for the resource you want to manage. For example, to manage an existing S3 bucket:
resource "aws_s3_bucket" "in-bucket-prod-0903"{
bucket = "existing-bucket"
}
- Run the
terraform import
Command:
Useterraform import
to connect the existing resource to your Terraform configuration. For example:
- To import an S3 bucket:
terraform import aws_s3_bucket.in-bucket-prod-0903 existing-bucket
- Check the Imported State:
Run
terraform show
to review the resource’s details in your Terraform state. This will confirm that the resource was successfully imported to your state file. By importing existing resources into Terraform:
- You gain version control for your infrastructure.
- You ensure consistent configurations across all environments.
- You simplify scaling and debugging by managing resources as code.
terraform import
is a powerful tool for bringing your existing resources under Terraform management. It links already created resources to your Terraform state, enabling you to manage them in the form of Terraform configurations.
Simplifying Terraform Workflow with Terrateam
Now as your infrastructure scales, coordinating security, compliance, and collaboration across multiple tools and teams becomes a bit challenging. Ensuring consistency and enforcing best practices across all the projects required too much manual effort, making it hard to scale.
This is where Terrateam comes into the picture. It brings all of these best practices together in one place, helping you to simplify your Terraform management, enforce security policies, and improve team collaboration. By integrating Terrateam, you can simplify your Terraform workflows, automate policy enforcement, and make sure that your infrastructure is always compliant and secure.
Here’s how you can integrate Terrateam with the best practices that we discussed above:
- Managing modules Modules in Terraform helps you organize and reuse your infrastructure as code, and Terrateam makes managing these modules much easier. It helps us track changes in module files, integrates them into our Terraform workflows, and makes sure that all the updates are securely planned and applied. By using Terrateam, we can collaborate on shared modules while maintaining control and consistency in the deployments.
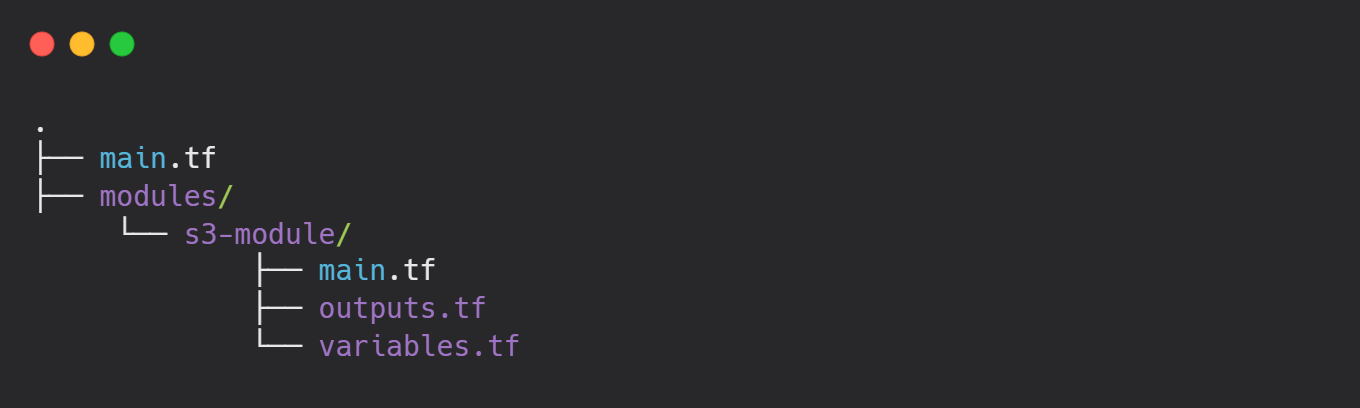
When you raise a pull request, Terrateam automatically provides a terraform plan
output within the same pull request. This allows you to preview the proposed infrastructure changes. After reviewing the plan output, you can comment terrateam apply
to execute the deployment. This workflow makes sure that all the changes to your infrastructure are reviewed and approved before being applied, maintaining a secure and collaborative process.
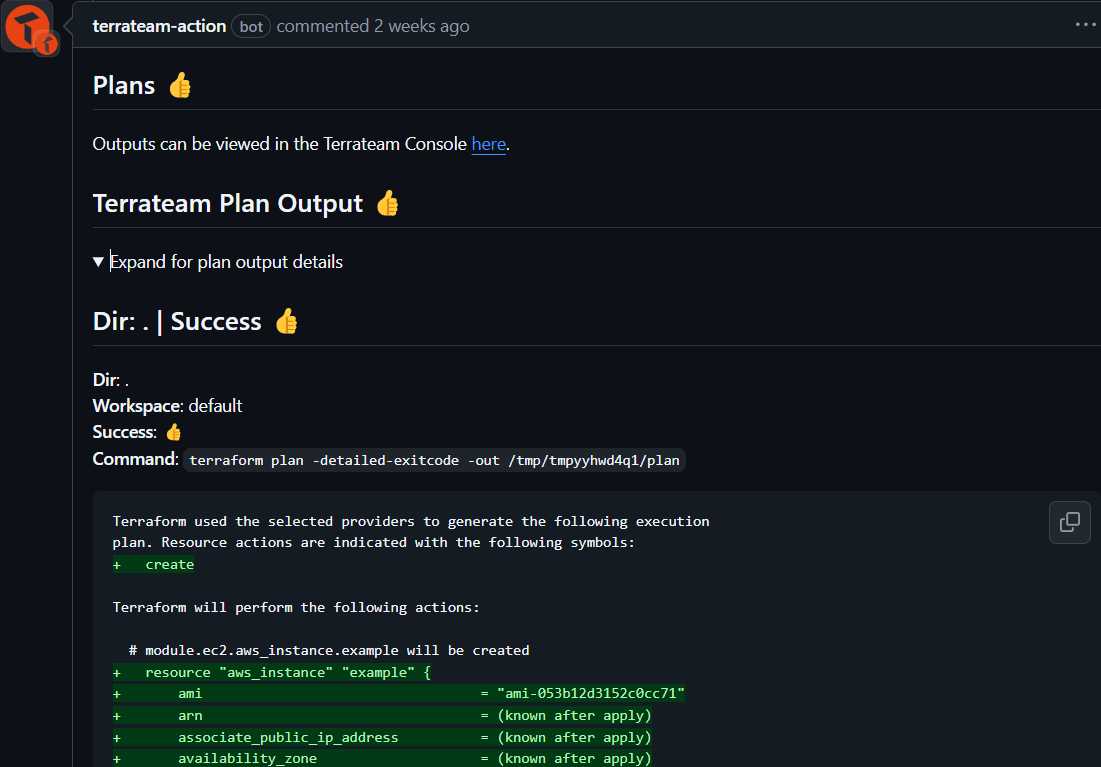
- Secure Secrets:
Terrateam provides multiple options to securely manage and use sensitive information, such as API keys and cloud provider credentials, in Terraform workflows. By using GitHub Secrets, environment variables, and tfvars files, Terrateam makes sure that your sensitive data is encrypted and securely stored. These values are only exposed during the necessary stages of the workflow, such as plan or apply, while maintaining strict security throughout the process.
To include a .tfvars file in your Terrateam workflow, you can specify its path using the extra_args
option. This setup applies the specified .tfvars file during the plan step, allowing you to customize Terraform variable values for a particular environment. For example:
workflows:
- tag_query: ""
plan:
- type: init
- type: plan
extra_args: ["-var-file=qa.tfvars"]
apply:
- type: init
- type: apply
This configuration enables you to define environment-specific values, such as database credentials, region configurations, or feature flags, in your .tfvars files.
- Use Remote Backends for State Management:
Using a remote backend like AWS S3 with DynamoDB locking is important for securely managing Terraform state in collaborative environments. Terrateam integrates smoothly with remote backends, ensuring all terraform plan
and terraform apply
operations use the same consistent state file, avoiding conflicts and deployment errors.
To configure a Terraform backend with AWS S3 and DynamoDB, first, create an S3 bucket (e.g., terraform-state-bucket
) using the AWS CLI and enable versioning (aws s3api put-bucket-versioning
) to track state changes. Next, set up a DynamoDB table for state locking (aws dynamodb create-table
) with a primary key named LockID
, making sure that it has sufficient read and write capacity to prevent simultaneous operations.
In your Terraform configuration, define the backend block, specifying the S3 bucket, DynamoDB table, and encryption (encrypt = true
). Use a unique key, like envs/prod/terraform.tfstate
, to separate environments. Finally, run the terraform init
command to initialize the backend, connecting Terraform to the S3 bucket and DynamoDB table to sync the state file and enable locking during operations.
terraform {
backend "s3" {
bucket = "terraform-state-bucket"
key = "envs/prod/terraform.tfstate"
region = "us-west-2"
encrypt = true
dynamodb_table = "terraform-lock-table"
}
}
- Automate Security Checks with Checkov and Terrateam:
Integrating Checkov into Terrateam workflows automates the detection of security misconfigurations within your Terraform configuration. This makes sure that every pull request and deployment passes through a strong security validation, reducing manual effort and minimizing risks.
Steps to Integrate Checkov into Terrateam Workflows
Terrateam is easily integrated with Checkov to scan Terraform plans for misconfigurations and security vulnerabilities before any changes are applied to your infrastructure. This automated process makes sure that each pull request is checked for issues such as open security risks or improper resource configurations.
To use Checkov in your workflow, add the checkov-wrapper
within your .terrateam/config.yml file. Each plan will then be automatically scanned for vulnerabilities and misconfigurations, reducing the risk of deploying insecure infrastructure.
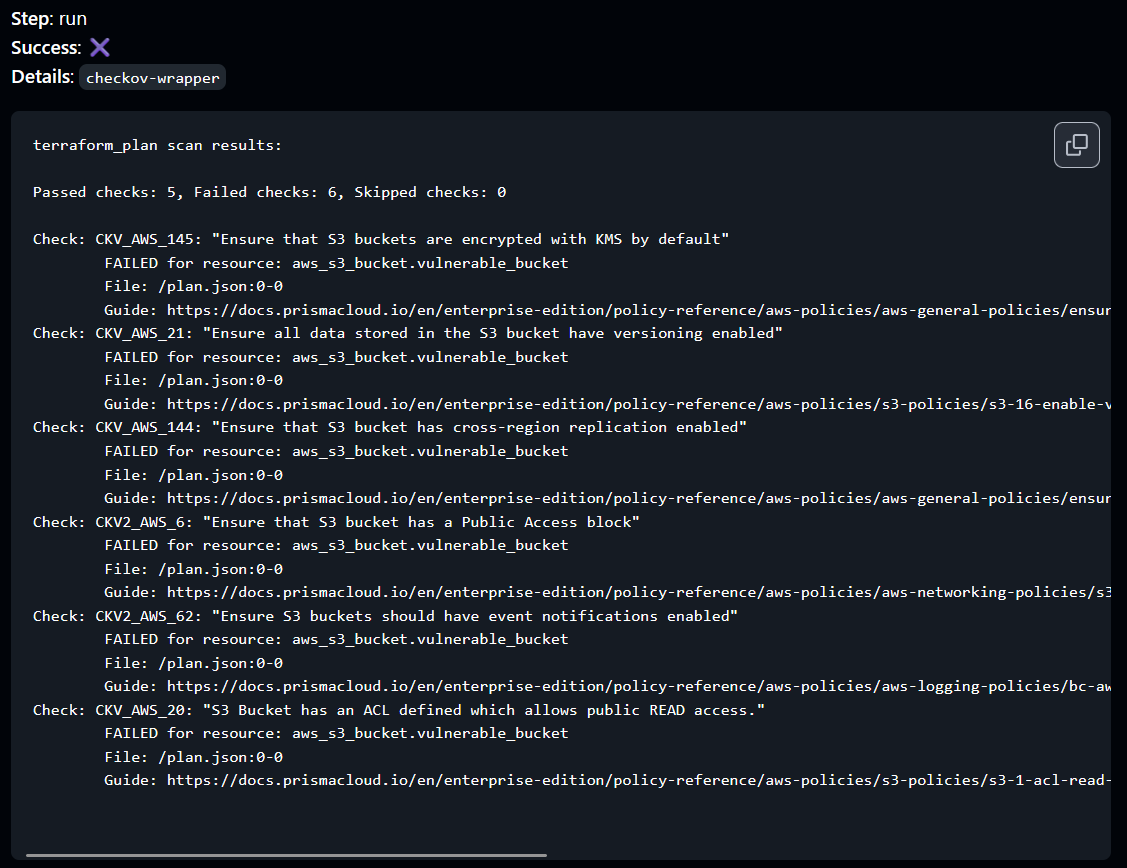
- Implement Role-Based Access Control (RBAC):
Terrateam’s RBAC feature simplifies access control for Terraform workflows by eliminating the complexity of setting up multiple configurations and permissions, as required by tools like GitHub Actions and OPA. With Terrateam, you can achieve granular access control using a single .terrateam/config.yml file. This allows teams to filter users and verify whether they have permission to perform specific Terraform operations, such as terraform plan
or terraform apply
, when raising a pull request. The configuration also enables organizations to enforce rules for approvals, ensuring that changes are reviewed thoroughly before they are applied to production environments.
For example, with a simple RBAC configuration in the .terrateam/config.yml file, you can allow all users to execute the terraform plan command while restricting terraform apply
to specific authorized users. In the following setup, any user can run terraform plan
to view proposed changes, but only the authorized user is permitted to apply those changes.
access_control:
enabled: true
policies:
- tag_query: ''
plan: ['*']
apply: ['user:Sid-Lais']
To see this configuration in action, start by creating a new branch within your repository and adding a main.tf file with your Terraform configuration. Open a pull request (PR) in your repository, and Terrateam will automatically enforce permissions based on the RBAC setup.
When a user initiates the terrateam plan
action, it will execute successfully, allowing them to preview the planned changes. However, if an unauthorized user attempts to run the terrateam apply action, the operation will fail, ensuring that only approved personnel can deploy infrastructure changes.
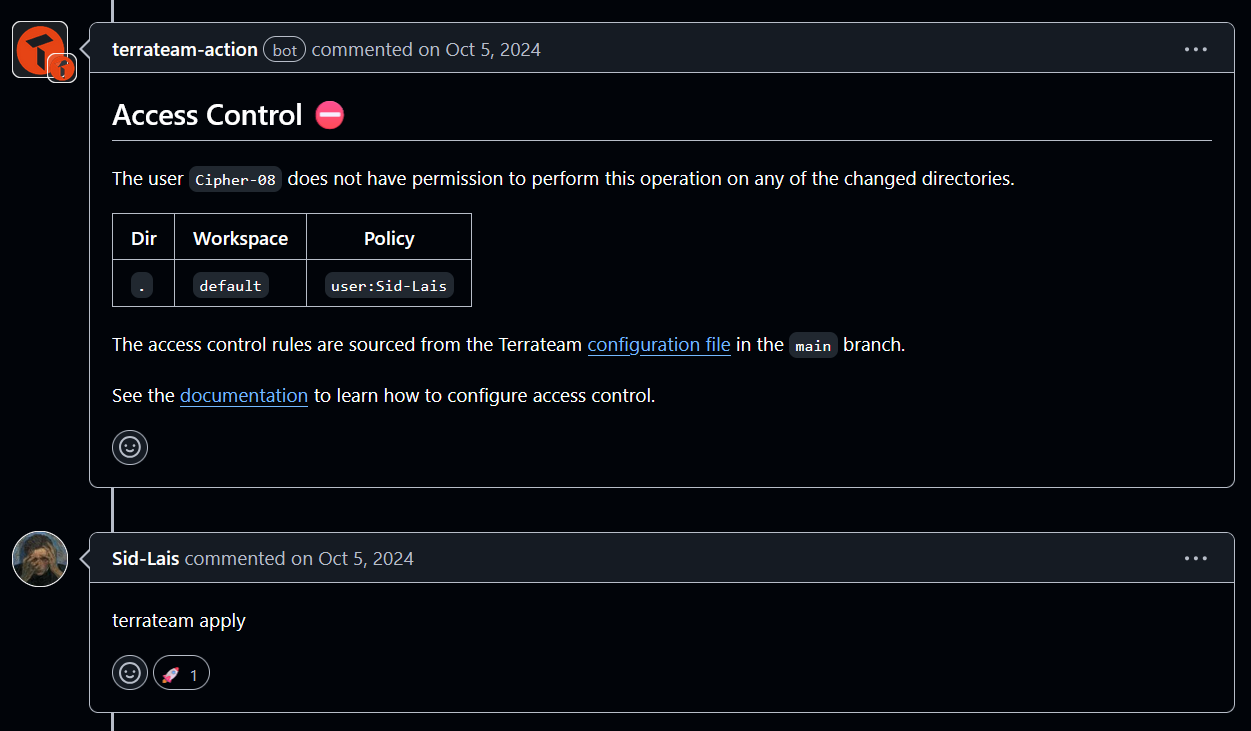
Without Terrateam, implementing access control often requires significant effort and complex configurations, particularly when managing granular permissions for multiple users or roles.
Conclusion
Using best practices for Terraform in CI/CD pipelines ensures smooth and reliable infrastructure management. Techniques like remote state management, automated security checks, and role-based access control reduce errors and improve collaboration, making scaling and maintenance easier.
FAQ
Q1. What are the challenges in the CI CD pipeline?
Common problems in CI/CD pipelines are slow builds, unreliable tests, inconsistent environments, weak security checks, and hard-to-debug errors due to poor logs.
Q2. How do you optimize a CI CD pipeline?
Speed up CI/CD pipelines by running jobs in parallel, caching dependencies, and removing unnecessary steps. Automate tests, deployments, and security scans, and regularly update pipeline settings for efficiency and consistency.
Q3. What are the secrets in CI-CD?
Secrets in CI/CD, like API keys or passwords, are stored securely using tools like GitHub Secrets or HashiCorp Vault. They are passed as environment variables to keep them safe and hidden from code or logs.
Q4. Where are Terraform workspaces stored?
Terraform workspaces are stored locally by default in the .terraform
directory within your project. When using remote backends, workspaces are stored in the backend (e.g., AWS S3, Terraform Cloud).
Q5. What is the difference between CI CD and pipeline?
CI/CD is the practice of automating testing, merging, and releasing code. A pipeline is a tool or process that carries out these steps, like building, testing, and deploying. CI/CD is the approach, and the pipeline is how it’s done.