Introduction to AWS Lambda with Terraform

Josh Pollara
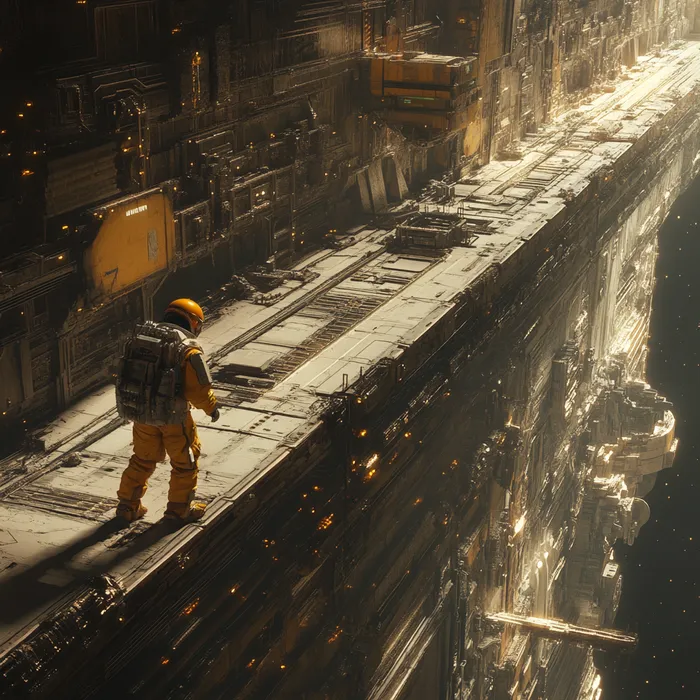
On this page
- Introduction to AWS Lambda with Terraform
- Overview of AWS Lambda
- How to manage and create AWS Lambda Functions using Terraform
- Creating Resources
- VPC
- Subnet
- Security Group
- IAM Role and Policy
- Python Lambda Function
- Packaging the Python Code
- Lambda Function
- Apply Changes with Terraform
- Monitoring AWS Lambda Functions
- Amazon CloudWatch
- Lambda Metrics with CloudWatch
- Using Terrateam to deploy Lambda Function
- Frequently Asked Questions
- Q. What are the 3 components of AWS Lambda?
- Q. Which runtime should I use with AWS Lambda?
- Q. How is AWS Lambda billed?
- Conclusion
Introduction to AWS Lambda with Terraform
AWS Lambda Functions have become an essential tool for developers who want to create and deploy serverless applications in the cloud. AWS Lambda allows code to execute in response to triggers without the need to manage servers, scale, or provisioning. When combined with Terraform, developers can automate and streamline the deployment process of their applications on AWS Lambda.
This blog post aims to introduce the integration of AWS Lambda Functions with Terraform, providing a comprehensive guide on deploying a Python application to AWS Lambda using Terraform. By the end of this post, you will clearly understand how to leverage Terraform and AWS Lambda to deploy serverless applications quickly.
Overview of AWS Lambda
In a serverless architecture, developers no longer need to concern themselves with the underlying virtual machines, nodes, or the tedious task of patching them. AWS Lambda automates the scaling, provisioning, and management of the infrastructure required to run the code, liberating developers to concentrate solely on crafting the functional aspects of their applications.
This reduces the complexity and eliminates the overhead associated with traditional infrastructure management, enabling a more streamlined and efficient development process.
AWS Lambda is a compute service that executes code in response to various triggers in a serverless architecture. These triggers can include HTTP requests via Amazon API Gateway, modifications made to objects in Amazon S3 buckets, updates to tables in Amazon DynamoDB, and more.
When an event matches the criteria set for a Lambda function, AWS Lambda automatically runs the code configured for that function. AWS Lambda manages this process entirely, ensuring high availability and automatic scaling to match the request rate.
Additionally, AWS Lambda supports various programming languages, including Node.js, Python, Java, Go, Ruby, and C#. This allows developers to use the language that best suits their application’s needs.
Creating and managing AWS Lambda functions consists of the following steps:
- Function code
Begin by writing your function code in a programming language supported by AWS Lambda.
- Package code
Compile or bundle your function code into a .zip file.
- Upload to AWS Lambda
Transfer this packaged code to AWS Lambda.
How to manage and create AWS Lambda Functions using Terraform
Deploying an AWS Lambda function with Terratform consists of the following steps:
- Terraform Code
Craft Terraform code to define and configure the Lambda function for deployment. Specify necessary details such as function name, runtime environment (e.g., Python), and other required settings.
- Create Lambda Function
Utilize Terraform to provision the AWS Lambda function based on the defined configuration. This step ensures the function is correctly set up within your AWS environment.
- Deploy Application
Once the Lambda function is created, link your Python application to it to deploy it within the serverless environment provided by AWS Lambda.
Following these steps can ensure that your serverless application is accurately configured and is ready to handle events within your AWS environment, which is managed through Terraform.
Post-deployment, you will be able to access multiple functions in your application using the Lambda function URL, which serves as a dedicated HTTP(S) endpoint for your function. This URL lets you directly invoke your Lambda function through standard web requests, simplifying the integration of your serverless application with other services or making it accessible over the internet.
Creating Resources
Terraform needs to know which AWS region to target when creating your Lambda function and related resources.
provider "aws" { region = "us-west-2"}
VPC
Create a new VPC or specify an existing cidr_block
below.
resource "aws_vpc" "example_vpc" { cidr_block = "10.0.0.0/16"}
Subnet
You must create or specify at least one subnet within the VPC where your Lambda function will operate. Subnets define a range of IP addresses in your VPC and can be configured to route traffic internally or externally.
resource "aws_subnet" "example_subnet" { vpc_id = aws_vpc.example_vpc.id cidr_block = "10.0.1.0/24"}
Security Group
Security groups serve as a protective barrier around your Lambda function, acting as a virtual shield, controlling inbound and outbound traffic. You’ll need to define rules that allow your Lambda function to communicate with other services.
resource "aws_security_group" "lambda_sg" { vpc_id = aws_vpc.example_vpc.id
ingress { from_port = 0 to_port = 0 protocol = "-1" cidr_blocks = ["0.0.0.0/0"] }
egress { from_port = 0 to_port = 0 protocol = "-1" cidr_blocks = ["0.0.0.0/0"] }}
IAM Role and Policy
Your Lambda function needs an IAM role with policies that permit it to access AWS services and compute resources. Lambda assumes this role when executing your function.
resource "aws_iam_role" "lambda_role" { name = "lambda_execution_role"
assume_role_policy = jsonencode({ Version = "2012-10-17", Statement = [{ Action = "sts:AssumeRole", Effect = "Allow", Principal = { Service = "lambda.amazonaws.com", }, }], })}
resource "aws_iam_policy" "lambda_policy" { name = "lambda_policy" policy = jsonencode({ Version = "2012-10-17", Statement = [{ Action = [ "logs:CreateLogGroup", "logs:CreateLogStream", "logs:PutLogEvents", ], Effect = "Allow", Resource = "arn:aws:logs:*:*:*", }], })}
resource "aws_iam_role_policy_attachment" "lambda_logs" { role = aws_iam_role.lambda_role.name policy_arn = aws_iam_policy.lambda_policy.arn}
Python Lambda Function
For simplicity of this demonstration, we will create a Python file named lambda_function.py
to deploy our Python application. This file contains a function that returns a simple Hello World!
. This example is designed to showcase the deployment process of a Python application using AWS Lambda.
However, it’s important to note that the deployment process would be identical if you work with Node.js, React, or any other programming language AWS Lambda supports.
import json
def lambda_handler(event, context): return {"statusCode": 200, "body": json.dumps("Hello World!")}
Packaging the Python Code
Zip your Python file, as AWS Lambda requires the source code has to be uploaded in this format.
For Linux :
zip lambda_function.zip lambda_function.py
For Windows :
Compress-Archive lambda_function.py lambda_function.zip
Lambda Function
Now, define your AWS Lambda function in Terraform, specifying its name, runtime, handler function, the IAM role it should use, and the path to the ZIP file containing your code.
resource "aws_lambda_function" "example_lambda" { function_name = "terrateam-function-b" handler = "lambda_function.lambda_handler" runtime = "python3.12" role = aws_iam_role.lambda_role.arn filename = "function.zip"}
In this example:
function_name
is set to “example_function”, giving your Lambda function a clear and identifiable name.handle
r is set to “lambda_function.lambda_handler”, indicating which function in your code AWS Lambda should execute.runtime
is set to “python3.8”, specifying the programming language and version your function is written in.role
uses the ARN of an IAM role named lambda_role, which you would have defined elsewhere in your Terraform configuration. This role grants your Lambda function the necessary permissions.filename
points to “function.zip”, the ZIP file containing your code and dependencies.
Apply Changes with Terraform
Deploy to your AWS account.
terraform initterraform validateterraform planterraform apply
We have created our function on the AWS console.
Following these steps, you’ve successfully defined an IAM role and policy, written a Python Lambda function, packaged the function, and deployed everything using Terraform. This approach allows you to manage and create AWS Lambda functions in a structured and repeatable manner, leveraging the power of infrastructure as code.
The next step is to create and configure a Function URL for your Lambda function. This will allow you to invoke your function directly via an HTTP(S) endpoint. Here are the steps to achieve this:
- Find the “Function URL” section on the function’s configuration page.
- Click on “Create function URL”.
- Choose the desired authentication method. For testing purposes, you might select “NONE” for no authentication. For production environments, consider using “AWS_IAM” for IAM-based authentication.
- Configure CORS settings if your function will be called from web applications hosted on different domains.
- Click on “Save” to create the Function URL. Following these steps, you’ve successfully deployed your AWS Lambda function using Terraform and created a Function URL.
You can now access your application via the function URL you provided. For example, my function’s URL is:
https://csheqrliddpe5t5k5eu7lpfkt40rckgc.lambda-url.us-east-1.on.aws/
You will get your URL displayed here :
Following these steps, you’ve successfully deployed your AWS Lambda function using Terraform and created a Function URL. Your application is now accessible and can be used by anyone with the URL.
Additionally, you can map your custom domain to this Function URL, such as lambda.terrateam.io
. This way, users won’t have to interact with the default AWS-generated domain, which can be challenging to remember and use.
Monitoring AWS Lambda Functions
Monitoring Lambda functions is essential for understanding their performance and behavior. AWS provides several tools, primarily Amazon CloudWatch, to help you monitor your Lambda functions.
Amazon CloudWatch
Amazon CloudWatch is an AWS service that monitors your AWS resources and applications running on the AWS platform. It is designed to collect and access all your performance and operational data in a standardized form:
- Metrics
- Logs
- Events
CloudWatch enables you to:
- Collect Metrics: CloudWatch collects metrics about your AWS resources, such as the number of function invocations, execution duration, and error rates from AWS Lambda.
- Set Alarms: You can create alarms that monitor your metrics for certain thresholds or anomalies, send notifications, or automatically change the resources you monitor.
- Visualize Data: CloudWatch allows you to create dashboards to visualize your metrics and understand your applications’ behavior.
- Store Logs: CloudWatch Logs allow you to aggregate, monitor, and store logs.
- Detect Anomalies: CloudWatch Anomaly Detection uses machine learning algorithms to detect anomalies in your metrics, helping you spot issues before they impact users.
- Automate Responses: You can set up actions to respond to state changes in your AWS resources.
Lambda Metrics with CloudWatch
AWS Lambda automatically reports a series of metrics to CloudWatch that you can use to monitor your functions. To view these metrics:
Acessing CloudWatch Metrics
Access Monitoring Tools: Once you’re in your Lambda function’s dashboard, look for the “Monitor” tab. Click on this tab to view a summary of the function’s metrics.
Open CloudWatch Console: In the “Monitor” tab, you will see several widgets displaying key metrics such as invocations, duration, errors, and throttles.
If you make any changes in your lambda function, such as creating a Function URL for your AWS Lambda function, the invocation requests and data will be logged in Amazon CloudWatch. Here are the steps to follow after making a Function URL to monitor and access logs:
- Navigate to the “Configuration” tab in your Lambda function’s dashboard.
- Look for the “Function URL” section and click “Create function URL”.
- Choose the appropriate authentication type and configure CORS settings if necessary.
- Once created, AWS Lambda automatically generates a unique URL endpoint for your function.
Use the generated Function URL to invoke your Lambda function.
Accessing CloudWatch Logs
- Open the Amazon CloudWatch console.
- Navigate to the “Logs” section and then to “Log Streams”.
- AWS Lambda logs show details of each invocation, including start and end times, execution duration, memory usage, and any errors or output generated by the function.
Using Terrateam to deploy Lambda Function
Terrateam is a powerful Terraform and OpenTofu GitOps CI/CD solution that seamlessly integrates with GitHub, enabling teams to deliver infrastructure faster and more efficiently. With Terrateam, you can easily manage your infrastructure as code, collaborate with your team, and automate your deployment processes.
After completing the setup guide and configuration of your Lambda function using Terraform, the final step in the deployment process involves integrating with Terrateam to automate the application of your Terraform changes.
- Create a pull request with your Terraform code and your Lambda zip file.
- Terrateam will automatically run a
terraform plan
and comment the output back to the pull request. - Comment
terrateam apply
to apply your changes and create your new AWS Lambda function.
This process not only automates the application of Terraform changes but also enforces best practices by requiring code review and approval before changes are applied, enhancing the security and reliability of your infrastructure deployments.
Frequently Asked Questions
Q. What are the 3 components of AWS Lambda?
The three components of AWS Lambda are:
- Function: This is the core component where you upload your code. It represents the code that AWS Lambda executes in response to events.
- Event Source: This component triggers the function. It can be an AWS service or a custom application that invokes your function.
- Configuration: This includes the settings and metadata for your Lambda function, such as the runtime environment, IAM roles, memory, timeout settings, and environment variables
Q. Which runtime should I use with AWS Lambda?
AWS Lambda is neutral to the choice of runtime. The choice of runtime is up to you.
Q. How is AWS Lambda billed?
Lambda is billing by number of requests and duration of requests. See AWS Lambda Pricing for details.
Conclusion
To wrap up, this guide has explained the basics of AWS Lambda, which helps you run code without worrying about servers. We’ve also learned how Terraform can make setting up and managing AWS Lambda functions easier. By using tools like CloudWatch for monitoring and Terrateam for deployment, we’ve shown how automation can make managing cloud resources smoother. This journey has emphasized how AWS Lambda, Terraform, and Terrateam work together to make managing cloud services easier and more efficient.